There have been a ton of web development frameworks and libraries over the years, but not many have caught on like ReactJS.
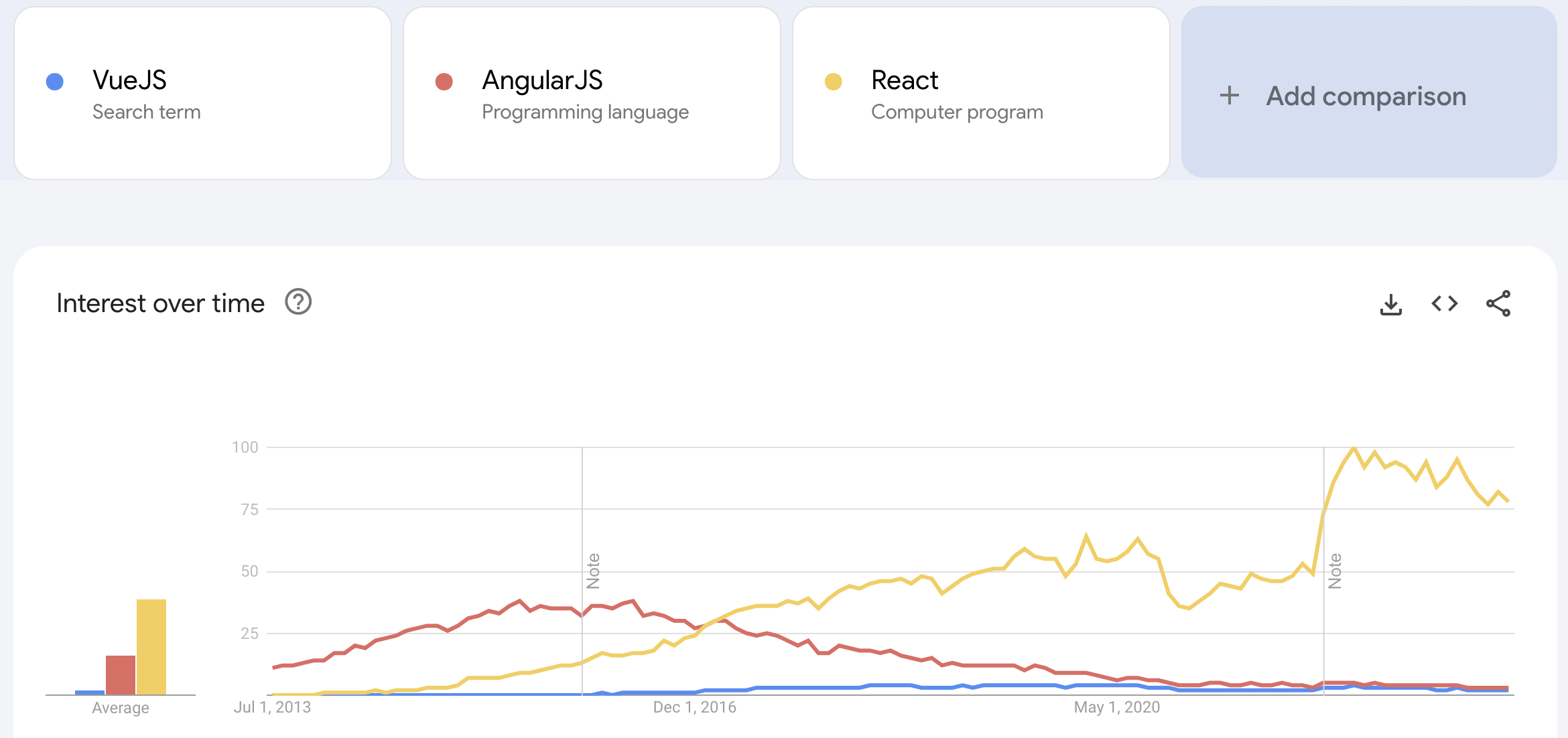
Originally released by Facebook in May of 2013, React has grown to be one of the most popular Javascript libraries in use today, has spawned a wide-ranging ecosystem of related libraries and tools and is estimated to be in use on just over 40% of the top 10,000 sites on the web today. Its usage is so widespread that there was even a full-length documentary produced about the origin story of React.
In this article, we’ll give you an overview of what ReactJS is, explore some of the tools and related libraries that make it even more useful and take a look at how you can use it on your next project.
Understanding ReactJS
When they were initially building ReactJS, Facebook was looking for a way to manage the complexity of their interface. Unlike many other Javascript libraries, React takes a declarative approach to building user interfaces. This means that instead of writing out all the steps necessary to create a particular interface, like you might do with DOM modification code in jQuery, you simply define for React what you want the user interface to look like with components and React creates it. Similarly, for any interface updates that need to be made, you declare what the new interface should look like and React re-renders the pieces of the interface that need to change.
In order to both take this declarative approach while avoiding un-necessary re-renders of an entire user interface, React uses the concept of a virtual DOM. The virtual DOM is a lightweight, in-memory representation of the actual DOM (Document Object Model) of a web page. When a change occurs in the application's state, React creates a virtual DOM to compare it with the previous state, identifying the minimal changes needed to update the real DOM. This process optimizes performance by reducing direct manipulation of the DOM, leading to faster rendering and improved user experience.
And because it was built for use in a large team, React is based on the idea of components, where each component can manage its own state as well as display properties and styling. This makes it easier to separate work into the various components of a project, makes it easier to keep track of which portion of the codebase is responsible for which pieces of functionality and keeps larger codebases much more organized.
Key ReactJS concepts
There are a few concepts that make React stand out from other Javascript frameworks that have come before and since.
Components
React is almost entirely component-based. React components allow the virtual DOM to work effectively, help developers keep their codebases more organized and enable component libraries that allow their team to re-use code. At its core, a component is a combination of markup, CSS and Javascript that dictates how a certain part of your user interface should be rendered. For example, this is how a Profile component might be implemented in React.
Once this component is built, anywhere you want to display a person’s profile picture, you can re-use this component. Components can even be nested inside other components, such as if you wanted to have a Gallery that contained multiple profiles.
JSX (JavaScript XML)
If you’re familiar with writing Javascript but some of the component syntax above is unfamiliar to you, that’s because React uses a syntax called JavascriptXML (JSX). JSX allows developers to write HTML-like code within JavaScript, simplifying the creation of UI components by seamlessly combining JavaScript logic with HTML structure. And while JSX provides a concise and expressive way to define UI elements and their behavior in a React application, the downside is that it needs to be transpiled to standard JavaScript before it can be understood by browsers. This extra step makes it a bit more complicated that writing vanilla Javascript, but truly helps unlock the power of components within a React application.
Props and State
One of the more difficult parts of building a Javascript web application is managing the state. For example, knowing which buttons have clicked, which options in a list are currently selected or how data should be flowing back and forth in the application.
React manages this by enforcing a one-way data flow from parent components to their child components. In this model, parent components pass data down to their children through properties, or props. When the data in the parent component changes, React efficiently updates the child components that depend on that data. Child components cannot directly modify the data received from their parent; instead, they communicate with their parent through callbacks or functions passed as props. This unidirectional data flow simplifies the debugging process, improves code maintainability, and enhances the performance by enabling React to optimize the rendering process effectively.
Using our example above, if we wanted the image URL and the alt text to be customizable for each instance of the profile component, we could use props.
Lifecycle Methods
Because of how React handles state and how data flows in a React application, it’s important to be able to execute code at specific portions of this flow. This is where lifecycle methods come in. Lifecycle methods are special functions that allow developers to hook into different stages of a component's life cycle. These methods provide opportunities to execute code at specific moments during the component's existence, such as when it is created, updated, or removed from the DOM.
One of the most common lifecycle methods is componentDidMount
, which is called immediately after the component is rendered to the DOM. This is where actions like fetching data for the component or manipulating the DOM usually occur.
In addition to lifecycle methods, React also provides Hooks, which serve as a replacement for various lifecycle methods by offering a more declarative and flexible way to handle side effects. Unlike componentDidMount
and other lifecycle methods that each have specific names and can only handle one side effect at a time, a Hook like useEffect
is used directly inside the functional component's body, allowing you to manage multiple side effects within the same component easily.
React Ecosystem
In addition to all of the features React provides out of the box, because it’s such a popular library, other libraries have been created around it to fill some of React’s gaps. Two prime examples of these sorts of add-on libraries are Redux and React Native.
Redux
React’s one-way data flow is very useful for managing data and state within an application, but there are some cases where that’s not what you need. Redux addresses several of the shortcomings of React’s state management by providing a single, centralized store that holds the entire application state. It’s generally more beneficial for larger and more complex applications with a significant amount of shared state and interactions between components.
In comparison to React’s out of the box state management, Redux gives developer teams a bit more structure when it comes to managing state, making it easier for multiple developers to collaborate on the same codebase. It also provides easy debugging with time-traveling, which means you can inspect and replay past actions and state changes to understand how the application arrived at a certain state.
React Native
Released only a couple years after React’s public release, React Native is a toolkit that allows developers who are comfortable building React applications to compile those applications into native mobile apps for iOS and Android. This means that, instead of having to maintain an entirely separate mobile codebase in a different language (Java for Android and Swift for iOS), you can re-use your React components that you have built for the web, while still getting the performance gains of a native mobile application. In addition, this unlocks mobile-specific features that you can’t access unless you build a native mobile application.
Originally, React Native was used to build the Instagram mobile application, but usage has expanded far beyond just applications inside the Meta ecosystem, with many popular brands using it to develop mobile apps.
Advantages and limitations of ReactJS
Given all of this, how should you decide if ReactJS is a good choice for your next project? Let’s take a look at some of the advantages and limitations of ReactJS to help you decide.
Advantages
- Enhanced performance with the virtual DOM: React uses the virtual DOM to only re-render components as necessary, giving it a performance advantage over many Javascript frameworks and libraries. Especially if you have an extremely complicated interface with many components or if you’ve been dealing with sluggish performance on the front end of your application, React might be a good choice.
- Reusable, component-based architecture: If your application has a component-based interface that could benefit from the reusable structure React provides or you have many developers on your team and need help keeping your code organized and avoiding merge conflicts, React’s component-based architecture can definitely be helpful.
- Strong community and vast ecosystem: Because React is so popular, a large community has built up around it. This means it’s easier to hire other developers to work with you, get support and help if you get stuck and take advantage of all the additional open source libraries that make React even more powerful.
Limitations
- Steep learning curve: Although there’s been a lot of improvement in simplifying React development in recent years, it can still be complicated for beginners to get started with, especially if you are new to Javascript.
- Initial setup and configuration: Compared to some other languages and frameworks, there is a lot more setup work that needs to be done with React before you can get started building the business logic of your application.
- Limited focus on templates and view-related tasks: ReactJS is first and foremost a front end framework, which means if you are having to build something that’s not front end related, you’ll likely need an additional tool. There’s nothing inherently wrong with this, as it’s what has made React good at what it does, but if you’re building a full stack application, you may want to consider how the backend tooling you will be using will integrate with a React front end.
Airplane as a platform for building your next ReactJS application
If you’re ready to build your next application on top of React, one of the tools that can help make this process even easier is Airplane. Airplane allows users to build powerful tasks and UIs using scripts, queries, APIs, and more.
The basic building blocks of Airplane are tasks, which are single or multi-step functions that anyone on your team can execute. Airplane Views is a React-based platform that makes it easy for engineers to build complex UIs within minutes. Airplane offers a rich React-based, pre-built component library, making it fast and easy for developers to build complex, and custom UIs. In addition to the pre-built component library, you can bring your own components or 3rd-party React components as well, making it a truly flexible platform.
To try out Airplane and build your first UI using React components in minutes, sign up for a free account or book a demo. If you are at a startup that is series A or earlier with under 100 employees, check out our Airplane Startup Program for $10,000 in Airplane credits for a year.