React is one of the most versatile libraries for creating robust frontend applications. It was launched in 2014, and many companies use it for their frontend application requirements. Additionally, over 44.3% of the respondents in Stack Overflow's 2022 Developer Survey responded that they work with React in a professional setup. With over two hundred thousand related job listings currently live on LinkedIn, React is a widely popular framework in the industry. As a result, having the ability to build React apps is a valued skill in the tech industry.
React apps are made up of components, and the components follow a predefined lifecycle once they are created. In this article, we'll walk through one of the most important lifecycle functions of React components, componentDidMount()
. We'll discuss its significance, how to use it, some of its common use cases, and what not to do with it.
React overview
React is commonly used in modern web development and is popular for its efficient performance and ability to easily deal with large data sets in memory. There are many other frameworks and libraries for building web apps in JavaScript, like Vue and Angular, but React's flexibility and lightweight nature have kept it ahead of the competition.
Some popular use cases for React when building web apps include:
- Building e-commerce websites and online stores
- Creating dashboards and data visualization tools
- Developing complex data-driven applications
Since its inception, React has relied on classes to build and compose components. Class-based React components define lifecycle functions like componentDidMount()
, constructor()
, render()
, and more to help users set up triggers based on the component's lifecycle. The componentDidMount()
function, in particular, is very useful when it comes to handling data fetches or DOM manipulation.
componentDidMount() overview
React has several component lifecycle functions that users can utilize to trigger reactions (or effects) at certain points in a component's lifecycle. Each lifecycle function has recommended use cases, and some functions like componentDidMount()
, render()
, and componentWillUnmount()
are quite common.
The componentDidMount()
component lifecycle function is called after a React component is mounted (or inserted into the DOM). So, at the time this method is called, the component is mounted and visible to the user. This method is useful for running operations in the React app that require the component to be present inside the DOM, such as setting up modals that need the size of the screen or position of the component in the DOM to calculate their attributes correctly.
However, since this function is part of the component lifecycle, we need to be careful when manipulating the component state from inside this function. An improperly implemented setState()
call in most lifecycle functions can cause the component's lifecycle to go in an infinite loop and ultimately crash the app or add performance overhead.
Common componentDidMount() use cases
The following are two of the most common use cases of the componentDidMount()
lifecycle method.
Loading data from an API
Most React apps rely on external data sources that are often accessed via REST APIs. Rather than loading all the data when an app starts, it makes more sense to allow each component to load its own data as it's rendered on the screen. This reduces the network and performance overhead that we'd encounter if all the data loaded upon starting the app.
Here's how we can use componentDidMount()
to load data from an external API:
Attaching event listeners
The componentDidMount()
lifecycle function is also often used to attach event listeners to components and objects that are present in the DOM. This is possible because this method only runs after the component is completely mounted and rendered in the DOM, providing access to the complete DOM object.
Here's how we can use componentDidMount()
to attach event listeners to the in-app objects:
Things to keep in mind when working with componentDidMount()
Now that we've walked through how to use this lifecycle function, here are a few points that should be kept in mind to avoid potential issues.
Avoid updating component state inside componentDidMount()
Although it's possible to update the component state from inside componentDidMount()
, it's recommended to avoid doing so as this might cause performance overhead in the app.
The setState()
call triggers a re-render of the component when it is called, which means that if we call setState()
from inside componentDidMount()
, the component will end up rendering twice. In most cases, we can set the initial state of the component in the constructor()
lifecycle function.
When we need to update the state of a component after loading data from a remote source (such as a REST API), we can call setState()
inside the promise or callback function of the data fetch operation. To update complex components like modals or tooltips, where the component needs to be mounted in the DOM before setting state values, we can call setState()
inside this function.
Cleaning up side effects using componentWillUnmount() to avoid resource leaks
A side effect is any interaction between a React component and the outside world. This includes making API calls to fetch data, setting up listeners or subscriptions, making changes to the DOM manually, and more.
It's crucial to remember to clean up or close any side effects created with componentDidMount()
when the component reaches the end of its lifecycle. Neglecting to do so can result in side effects existing independently of the component and consuming host resources.
Here's an example demonstrating how to clean up API calls made in componentDidMount()
:
Note: Each operation performed in the componentDidMount()
function will have its own way of being canceled or cleaned up in the componentWillUnmount()
function. Removing an event listener is different from canceling a request, and we'll need to use the appropriate method for the operation for optimal performance.
Not using componentDidMount() in server-side rendered apps
As we saw in previous examples, the componentDidMount()
function is specific to the client side of an application, as it runs at the end of the React component lifecycle (which only initiates when the app is loaded on the client side).
This means that we shouldn't use the componentDidMount()
function if we're working with a server-side-rendered application where the React components will be rendered on the server before their HTML is sent to the client. On the server side, the componentDidMount()
function will not be called, and any API calls defined inside it will not be executed.
If the component is dependent on its lifecycle methods, we should use ReactDOM.hydrate()
on the client side. This will ensure that the necessary listeners are attached to the pre-rendered HTML components and enable the component lifecycle to run normally.
How many times does componentDidMount() run?
The componentDidMount()
lifecycle function runs when the component is mounted onto the DOM, which means that it will run as many times as the component is mounted. In normal use cases (i.e., for components that are mounted once and then updated via state changes), this lifecycle method is only called once. We can see it in the code snippet below:
Here's what the console would look like when the app is loaded:
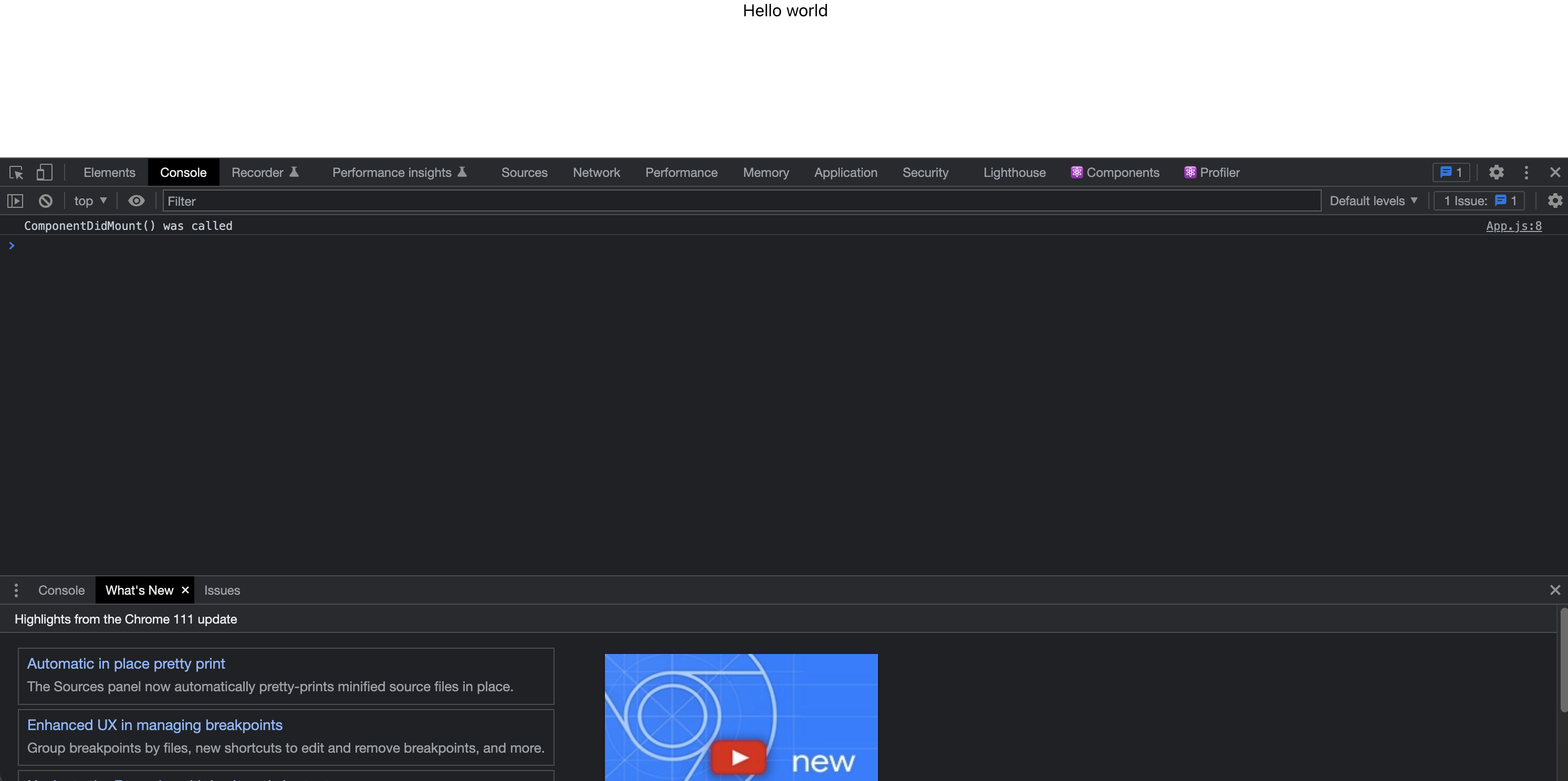
However, if we use a special prop called key
when rendering the component and change its value using the parent component state, the component will unmount and then remount, retriggering the componentDidMount()
function. The key
prop is often used when working with dynamic lists in React. It's important to be aware of its effect on the lifecycle of the components so that we don't end up creating components with erratic behavior. The important thing to remember here is to avoid changing the key
value unless it's necessary, and if we do, we must make sure to handle the unmount and remount of the component in the code.
In the following example, we can see the key
prop in action, retriggering the componentDidMount()
function whenever it is updated:
We can now see that the same line is printed twice after the five-second timer fires and updates the key:
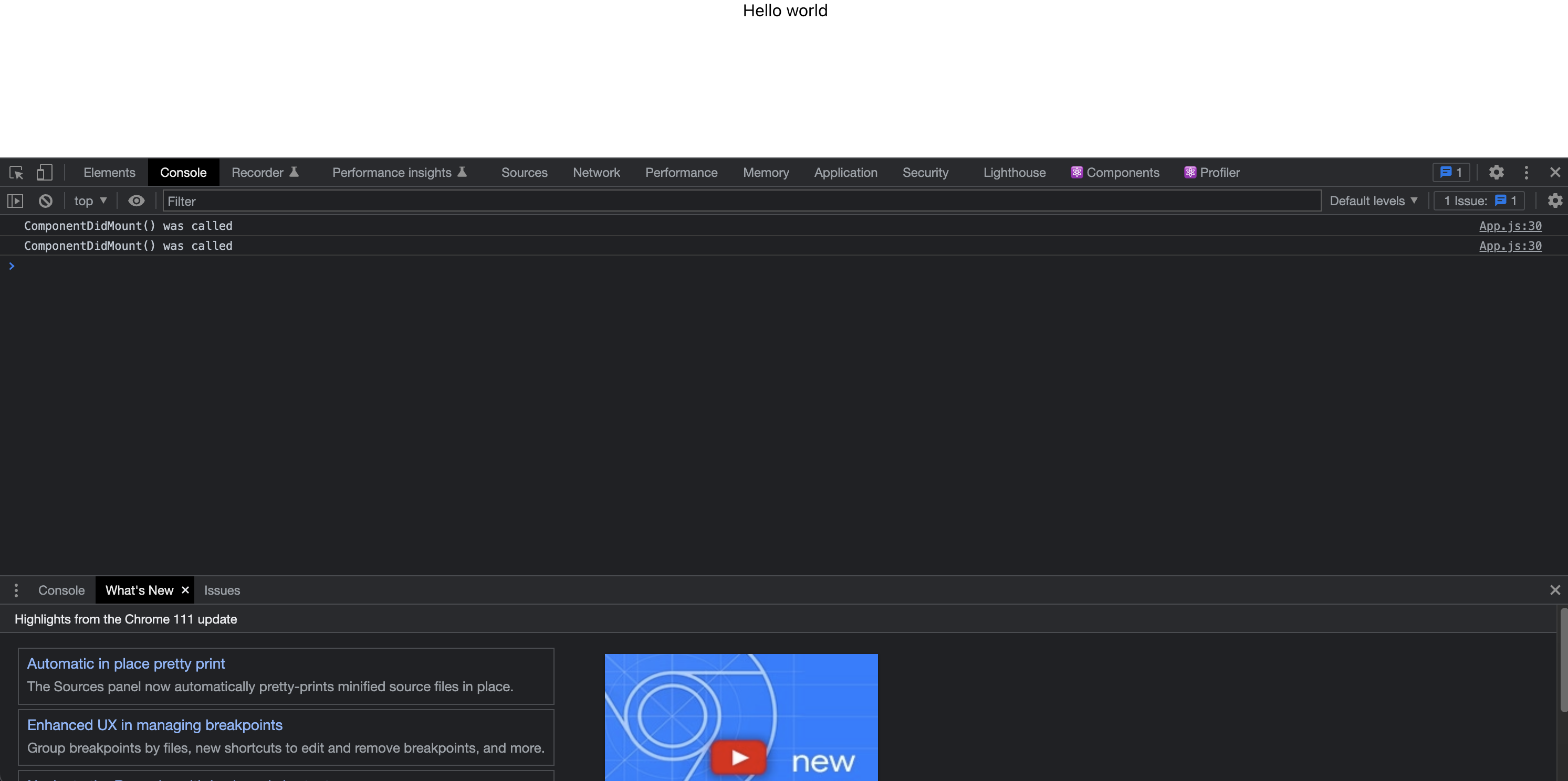
Introducing Airplane Views: Build React-based UIs quickly
Airplane is the developer platform for building custom internal tools. You can transform scripts, queries, APIs, and more into powerful UIs and workflows within minutes. The basic building blocks of Airplane are Tasks, which are single or multi-step functions that anyone on your team can use. Airplane also offers Airplane Views, which is a React-based platform that makes it easy for users to build custom UIs in minutes.
Airplane Views offers an extensive template library and component library, making it easy to start using it. Users can also build custom components or utilize third-party components easily.
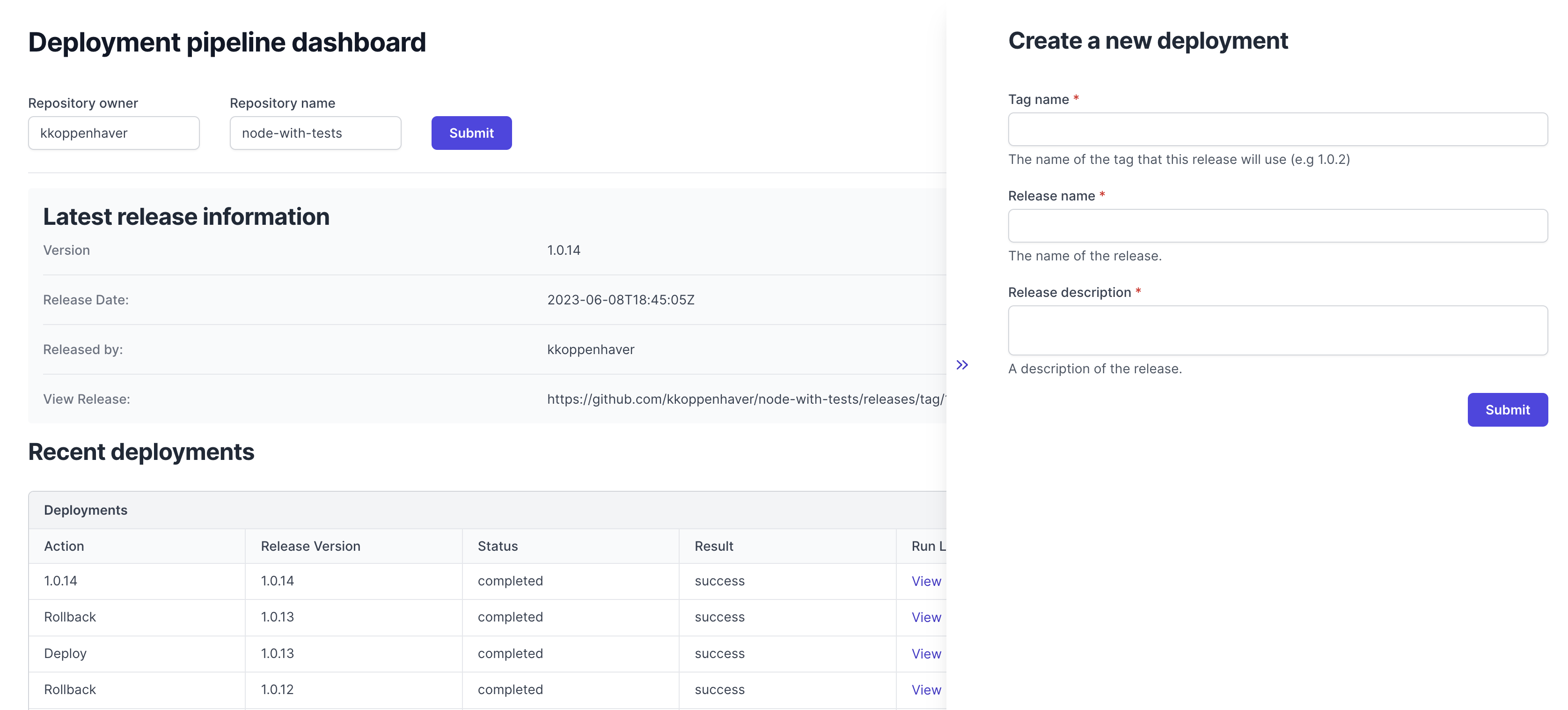
Airplane also offers strong built-ins, such as permissions setting, audit logs, job scheduling, and more.
To try it out yourself and build your first UI in minutes, sign up for a free account or book a demo 👋. If you are at a startup that is series A or earlier with under 100 employees, check out our Airplane Startup Program for $10,000 in Airplane credits for a year.