In this article we'll discuss six ways to leverage feature flags to boost productivity and walk through how to build a lightweight feature flag in under 5 minutes.
What are feature flags
In software development, feature flags describe the process of enabling or disabling an application feature during runtime without manually deploying new code. For example, you may want to enable certain features for specific enterprise customers but not for other users. You can also use feature flags to deactivate a feature for development or for testing purposes.
One perk of feature flags is that they enable you to make changes without publishing more code. This approach gives us greater control over the product lifecycle, making it easier to rapidly iterate if something goes wrong.
We can implement feature flags in a variety of ways. For example, it’s common to use SaaS platforms like LaunchDarkly, Split, or Optimizely to enable feature management.
In an application setting, feature flags enable us to alter our application’s behavior without changing the code. However, for feature flags to work in applications, we must pre-ship the features we wish to toggle or flag alongside a conditional block. This conditional block enables us to render this feature when the need arises:
Additionally, depending on how we want to manage our features, we'll probably want a platform that lets administrators toggle features easily from a UI.
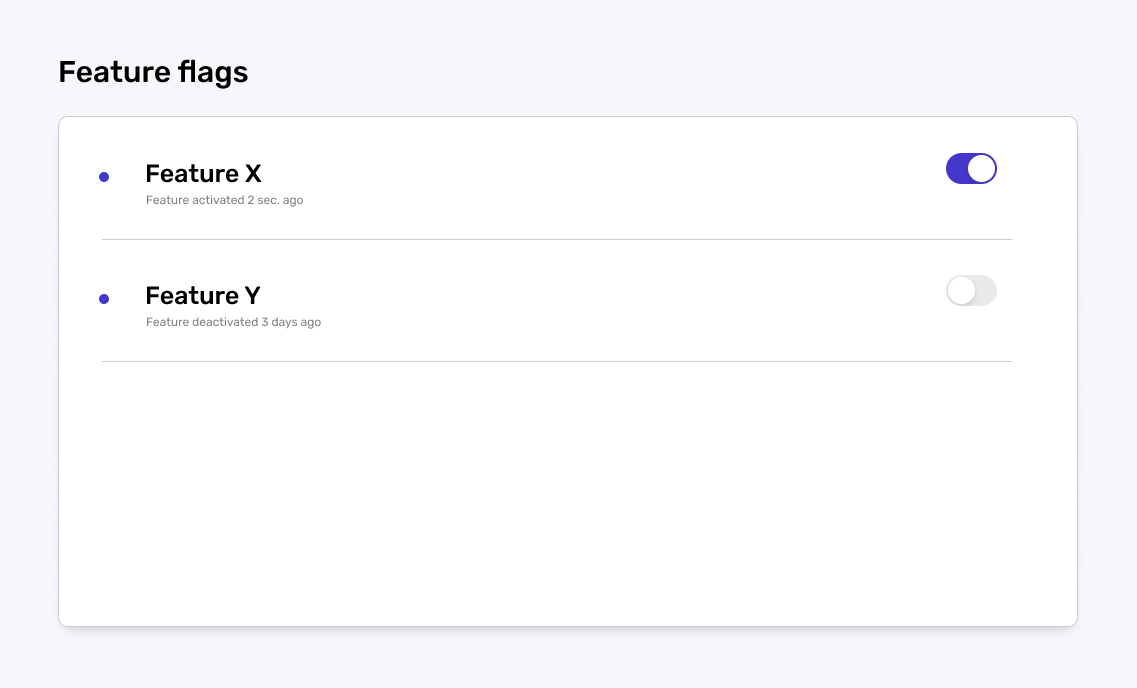
Six ways to use feature flags to boost productivity
A huge benefit of feature flags is that they enable us build more quickly. We can work on multiple features at once and overall, we are empowered to iterate and release new versions more often. We've outlined six ways to use feature flags to boost productivity below.
Soft rollouts
A soft rollout is a test release of a feature or service to a small group of people before it is fully available to the general public. Companies typically use soft rollouts to gather data and customer feedback and to gauge widespread acceptance of a feature before making it public. We usually release application features deployed in this way with little to no marketing, allowing us to focus more on product development.
Feature flags for soft rollouts enable us to pursue continuous deployment by gradually rolling out a feature to a subset of users. We can publish features that aren’t immediately active, then turn them on without republishing code.
Additionally, testing in smaller increments first helps detect bugs earlier and minimize our risk of errors.
Enabling non-developers to safely manage features for customers
Even when regular updates are required, we don't want to share project source code broadly because even small (unintentional) tweaks can result in security breaches and other issues.
At the same time, however, we may want non-technical teammates to have the ability to update features for customers. Feature flags give us the ability to enable non-developers to safely make modifications like this without giving them access to the production database directly. For example, we could give the marketing team the ability to set up segmented campaigns without relying on engineers. Another use case is providing customer support reps with the ability to grant temporary access to enterprise features or provide discounts.
When used effectively, feature flags can help technical and non-technical team members save time and operate securely.
Testing
Feature flags can also assist with basic user research. For example, consider the A/B testing process.
In A/B testing for UX research, an administrator turns on feature A and gathers feedback and opinions from users. Then, the administrator soft-rolls out feature B for different users to collect the same type of feedback. The administrator then uses the feedback from both features to determine which feature to release.
Feature flags support conducting A/B tests by enabling us to roll out a feature to a certain percentage of users and receive their feedback before releasing the feature publicly. We can discover bugs or other issues before they enter production, saving us time and resources in the long run.
Data migrations
Feature flags can also help us when we’re migrating data. This process might move data from one database to another or transform the data form entirely.
Suppose we have a user list we’d like to import into a new system. Normally, this would require downtime since data migration code would need to execute before the rest of our users could access the application. However, feature flags enable us to choose when to activate the data migration without shutting down the server.
While the technical procedure for this differs based on developer decisions, let’s consider an example:
Let’s say an organization launches a new service and migrates user data to a new storage system. This new service largely overlaps with the old one but has some new UI elements that interact with the new data storage system. The organization wants to demonstrate the updates to users whose data has been migrated. They do not, however, want users whose data has not yet migrated to have access to the new UI.
To ensure users view the correct UI, the organization can wrap the new design elements in a feature flag that checks whether a user’s data has migrated successfully. If it has, it renders the updated UI. Otherwise, it renders the old service.
Here’s some pseudocode to demonstrate the implementation:
Feature flags can also be used to simplify testing apps using live data for a portion of a user base. They can facilitate rolling back to previous editions of this data in the event of a compromising incident.
Handling system issues
Feature flags are a suitable tool when dealing with system issues. While outages and errors are inevitable, feature flags can help you provide an effective response.
For example, we can set an error dialog or page to toggle on when outages occur. We might even place part — or all — of our application into maintenance mode.
The implementation process for this is pretty straightforward. In most cases, we’ll have a channel or some kind of API that exposes the maintenance toggle status.
In our main application, before the application is rendered, we check the maintenance toggle state and act accordingly. Here’s a snippet of pseudocode for this process:
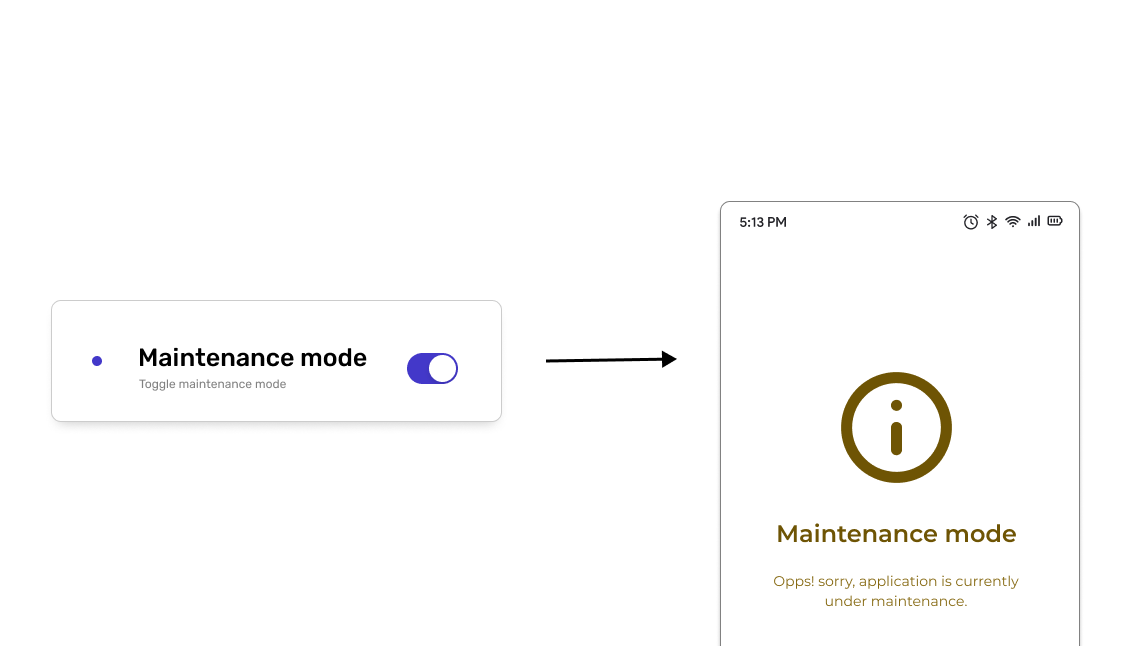
Multiple user experience
We often want to serve different app versions to distinct user groups and can use feature flags to do so.
For example, we can use feature flags to provide a simplified version of our site for mobile users. Alternatively, we might offer a different user experience depending on the user’s subscription tier.
We can also use feature flags when granting administrative privileges to some users while denying these privileges to others. For example, a SaaS company might want to enable single sign-on (SSO) only for accounts that have paid for that feature. Feature flags are an easy way for us to achieve this.
With a feature flag, we can provide various experiences to different people without compromising quality or security.
Now that we've covered some ways to use feature flags to boost productivity, let's explore a platform that can help us build them out - Airplane.
Build a feature flag in under 5 minutes with Airplane
Airplane is a developer platform to quickly turn scripts and queries into secure, internal applications. You can use Airplane for things like runbook automation, scheduled operations, customer onboarding, and more.
While Airplane isn’t a dedicated feature management system like LaunchDarkly or Split, a lot of teams leverage Airplane for feature flags when they have simpler needs that don't require a heavyweight solution (and because you can use Airplane for many other internal tooling use cases). For example, Airplane will provide the quickest way to implement something simple to turn on and off certain features for specific customers.
Later on, if you decide to adopt a dedicated feature management platform, Airplane supports an easy integration. You can use Airplane to hit their API while using Airplane for much more granular permission management, audit logging, and approval flows.
Create a feature flag using Node
Let's build a feature flag using Airplane tasks. In Airplane, a task is a lightweight app that represents a single business operation that you want your team to be able to execute. We'll create a simple task — a Node.js script that allows us to send a parameter with which we can toggle our "maintenance mode" on and off.
Start by signing up for a free Airplane account. Then, click 'New task' to create a new Node task. Note that in this example we'll be using the UI to create our task but we can also create and manage tasks entirely in code using the CLI (left side of the below screenshot).
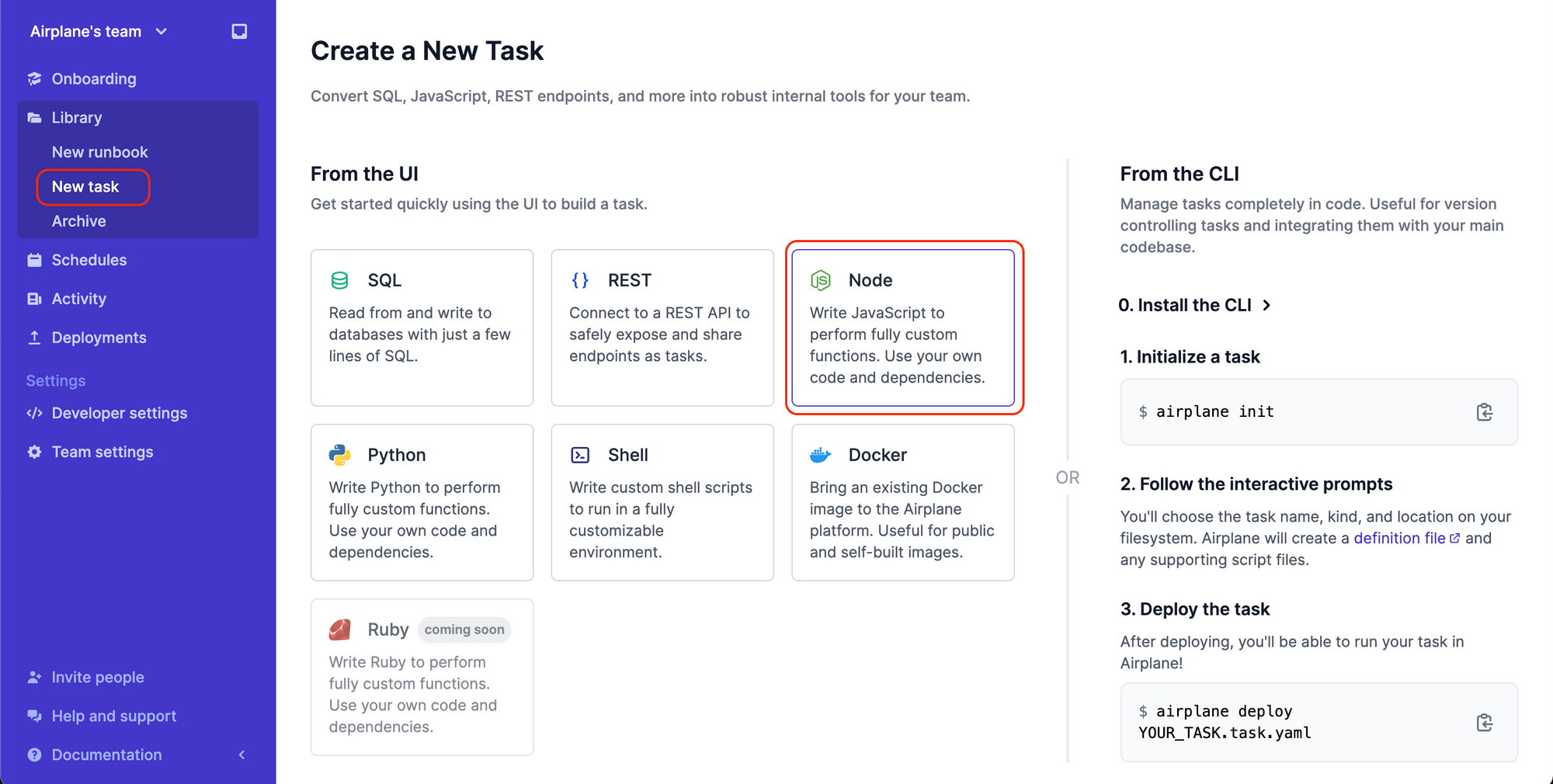
Selecting the Node option requires us to choose the version of Node we want to use for running our task. Once that’s done, we can name our task and define its parameters - which will allow you to prompt for user input.
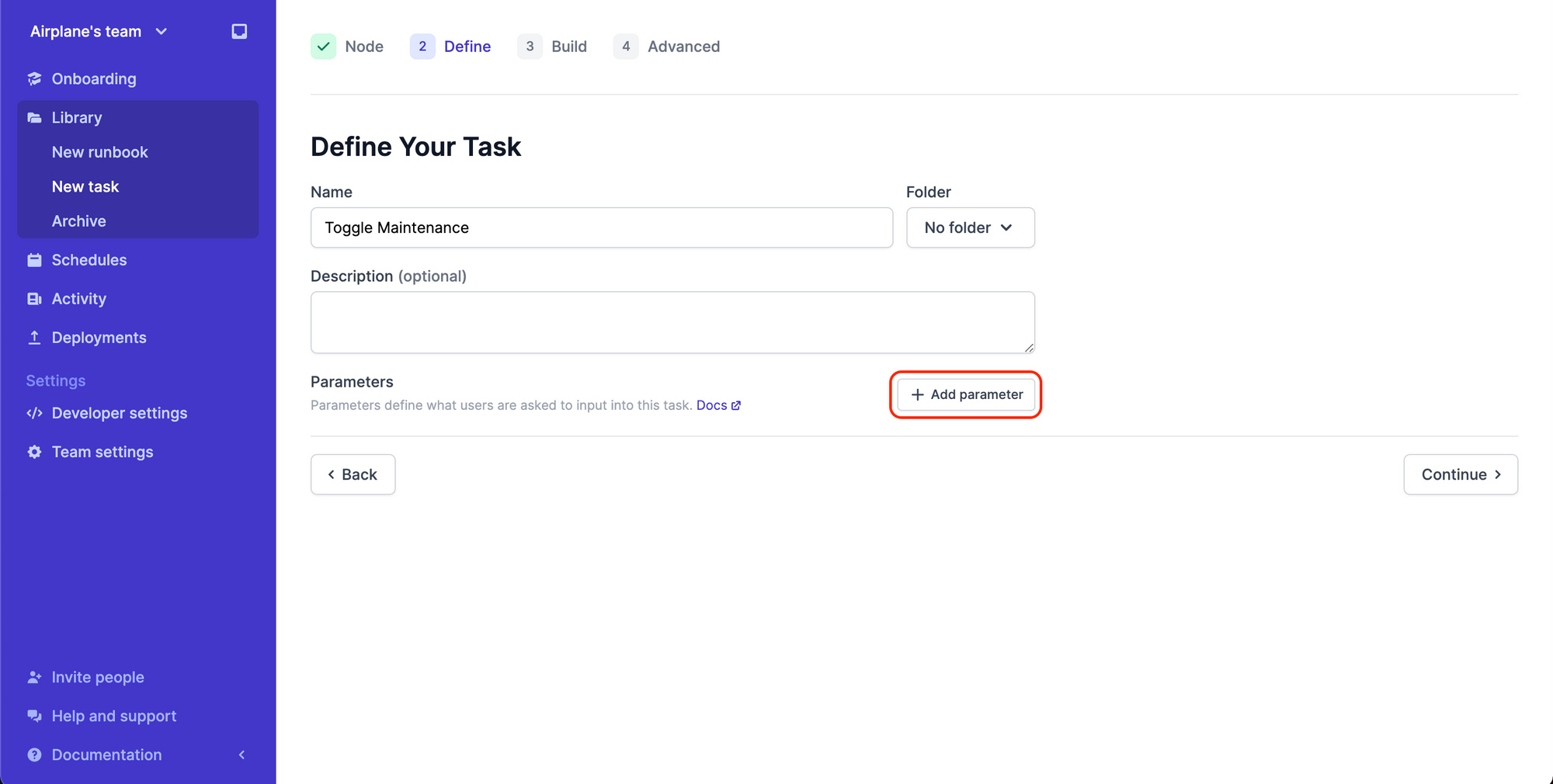
As shown in the screenshot below, we've named our task "Toggle Maintenance" and added a parameter of the same name that accepts a boolean (true/false) value. When we need to use our feature flag, we'll toggle this parameter to activate/de-activate maintenance mode.
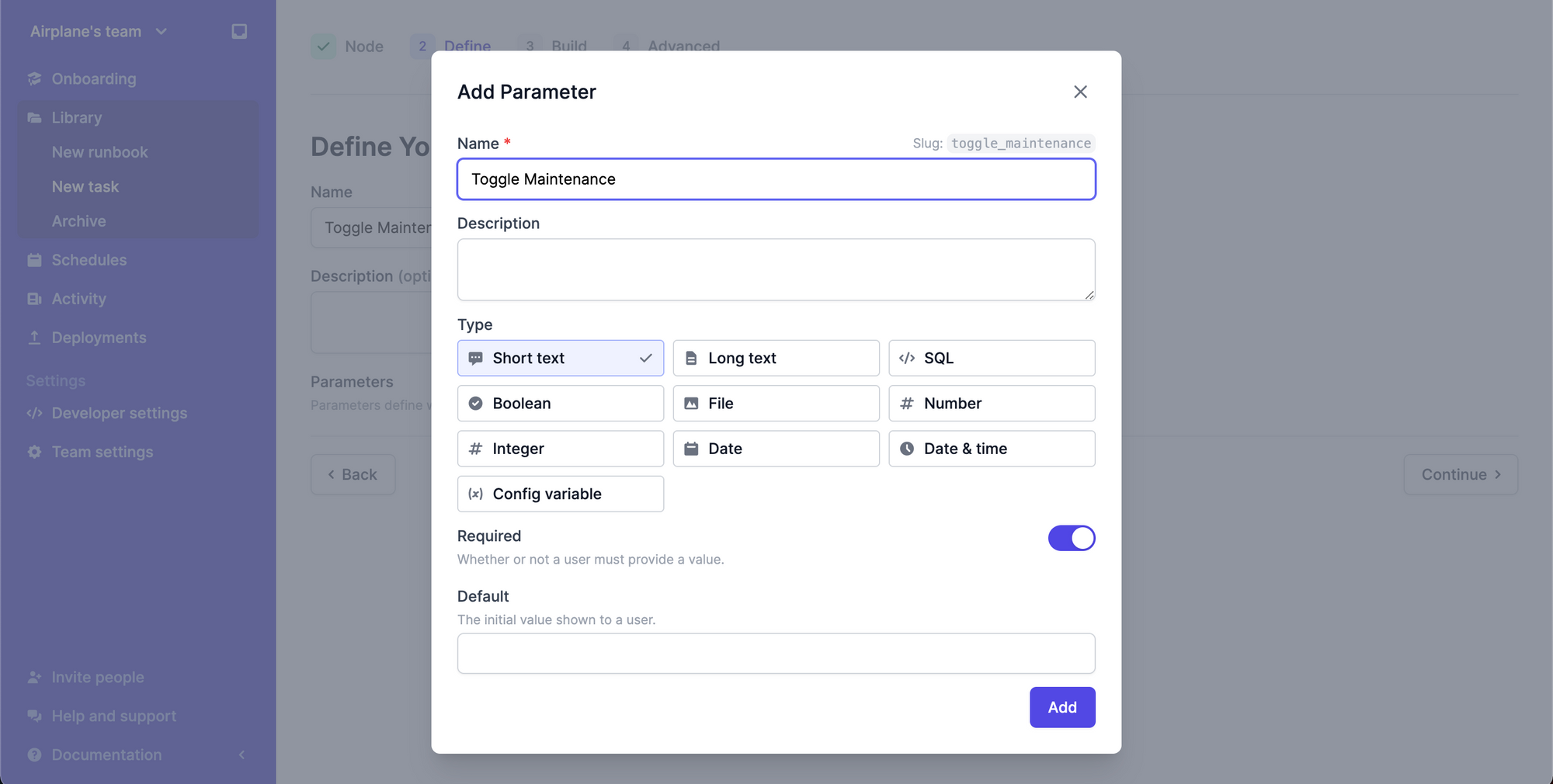
Once we've created our task, we'll see a quick set of instructions on how to start writing the script for our task locally.
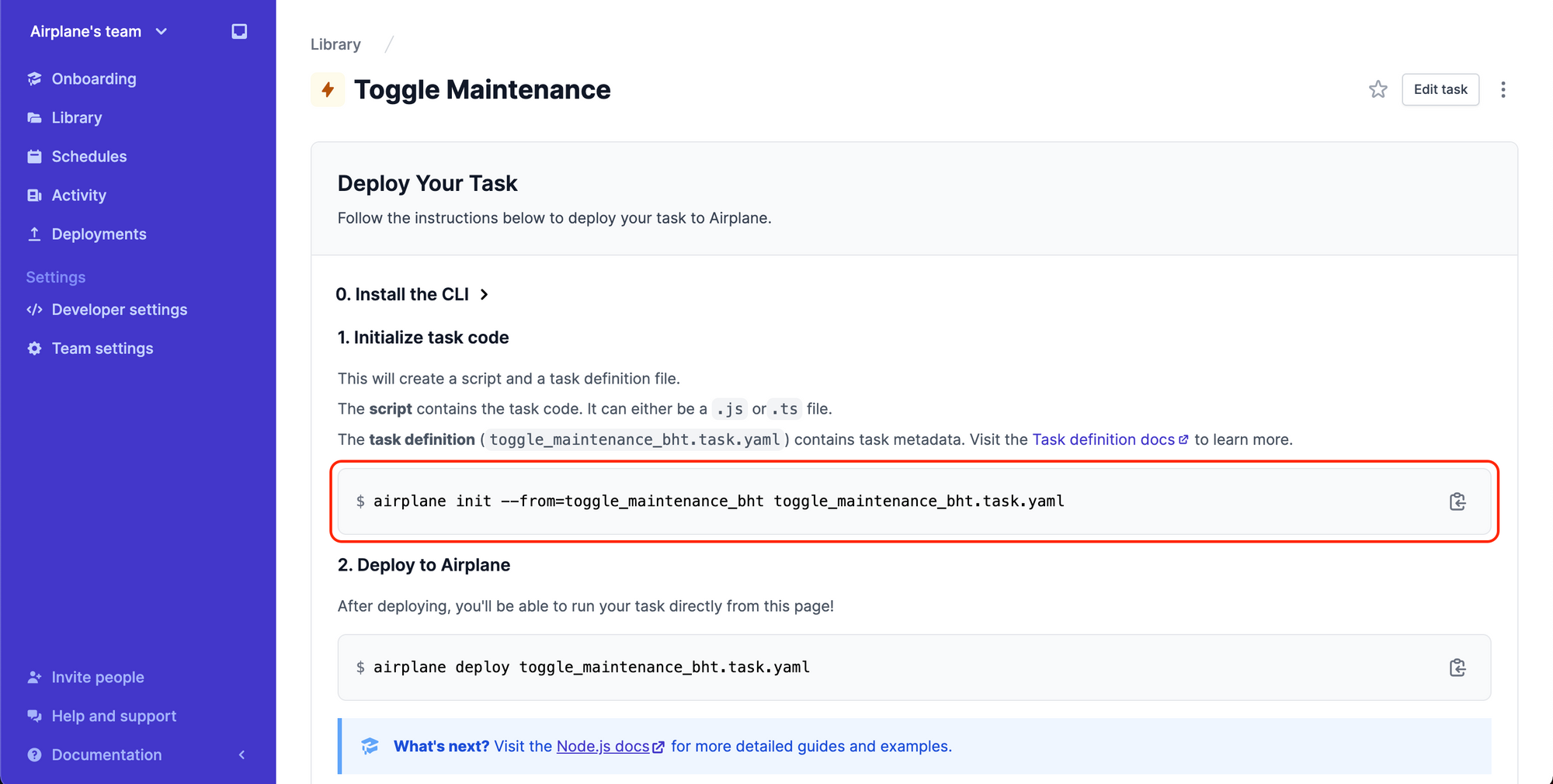
In this example, we'll run the following command:
airplane init --from=toggle_maintenance_bht toggle_maintenance_bht.task.yaml
Running this command will create two files: toggle_maintenance_bht.js
and toggle_maintenance_bht.task.yaml
. The first file contains the main code for running our script and the second is a definition file that describes the structure of our task.
To continue, let's open the first JavaScript file and replace its contents with the following code:
With this code, we’ve defined a new variable, toggleMaintenance
, that retrieves the boolean parameter that we set up earlier and then sends a request to an imaginary API to change the application's maintenance mode state. In addition, for feedback purposes, we've returned the API response in our task.
Please keep in mind that querying an API request in this type of request will necessitate authenticating the request sender with some sort of token. In order to keep this example concise, we've avoided including that here.
Once all this is completed, we can run this task locally with the following:
airplane dev toggle_maintenance_bht.task.yaml
or deploy it to Airplane using airplane deploy toggle_maintenance_bht.task.yaml
.
Once we deploy our task to Airplane, the task will automatically update with the following web form in the UI.
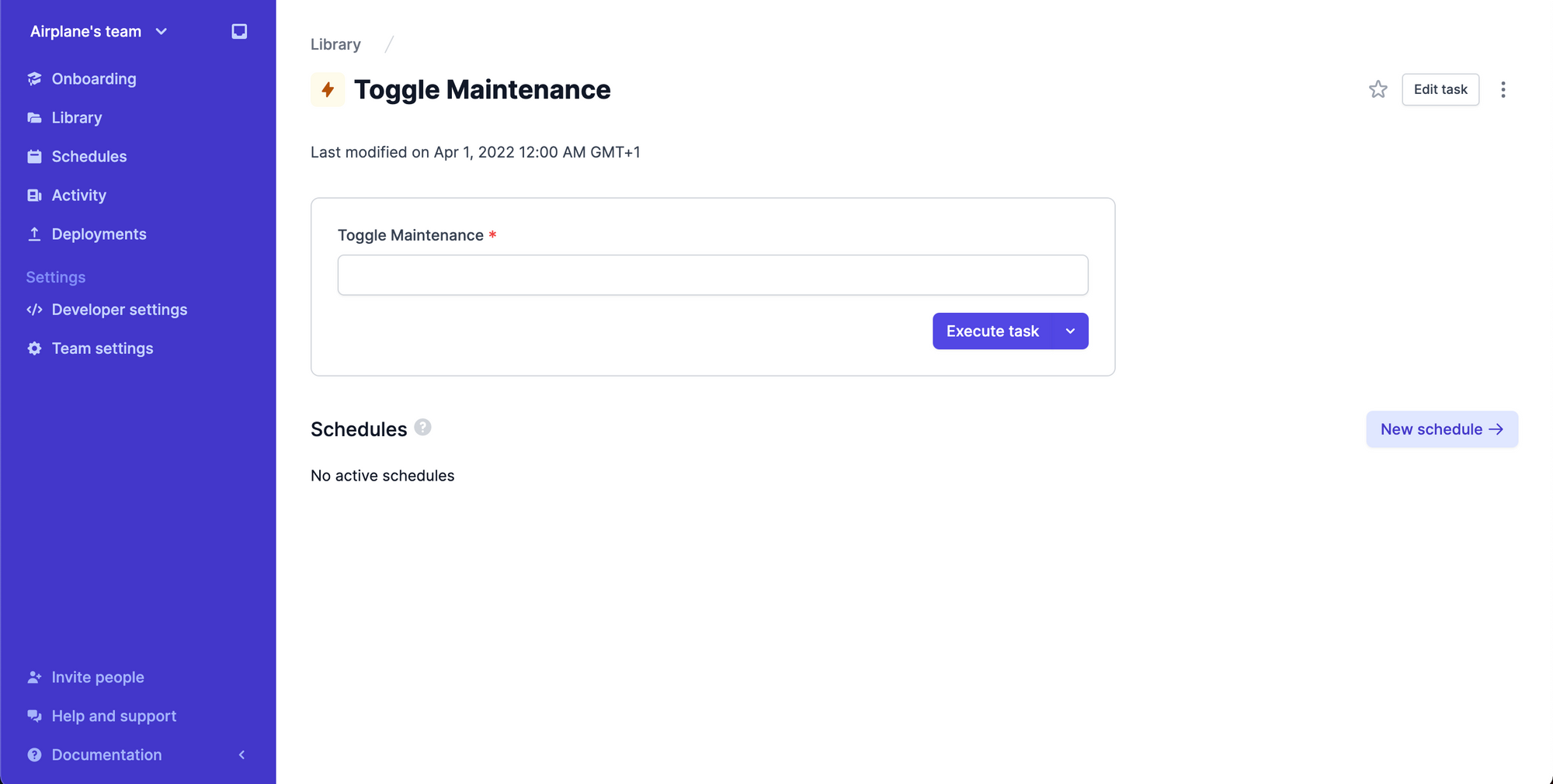
That's it! That's how you can create a lightweight feature flag in Airplane.
As we've covered in this article, feature flags allow us to modify the state of an application feature during runtime without explicitly deploying new code. We can use feature flags to increase productivity in many ways, including implementing soft rollouts, easing data migrations, handling system issues, and more.
Using Airplane, you can build lightweight feature flags in minutes. You can also use Airplane to transform your scripts and SQL queries into robust, internal apps that anyone can use for all sorts of use cases from automating customer onboarding to enabling sensitive operations to monitoring and alerting on database issues.
You can check out more use cases on our Examples gallery and sign up for a free Airplane account to get started.