Filtering records on the client side is a common practice, especially with data-driven applications. With client-side filtering, users can search, sort, and narrow down the data displayed on the user interface based on specific criteria without making additional server requests. It provides a more responsive and interactive user experience.
There are several advantages to performing client-side filtering, including:
- Reduced server load: Performing filtering on the client side helps offload the filtering logic from the server. This reduces the number of requests made to the server, which in turn helps improve the overall performance of the application, especially when dealing with large data sets or high user traffic.
- Faster response times: Filtering records on the client side allows for instant filtering and immediate feedback to the user. The user does not have to wait for server responses, resulting in faster response times and a smooth user experience.
- Offline filtering: Client-side filtering allows users to filter the available data even when their device is experiencing connectivity issues.
In this article, we’ll walk through the various methods for filtering data in a React application. Additionally, we’ll see how to preprocess data using the map()
and reduce()
functions before rendering it in the user interface.
How to filter records in React
In this section, we'll filter records in React by creating an application and applying various filtering methods using a set of local data. To follow along, ensure you have the following:
Creating a React application
Execute the following command in the terminal to create a React application using Vite:
The command above works for npm version 7+. If you have a lower version of npm, use npm create vite@latest react-filter-features --template react
.
This command creates a React application named react-filter-features
using Vite as the build tool. Navigate to the newly created folder and install the required dependencies using the commands below:
We can verify that everything works as expected by executing the command npm run dev
in the terminal to start a development server and then opening http://localhost:5173 in the browser.
Demonstrating the various possible methods with code examples
Now that the React application is ready, we can implement various methods for filtering through records.
Using the filter() function
We’ll first use the filter()
function on an array of primitive data types. We’ll then use the same function with a custom handler on an array of custom object types with single or multiple filter conditions.
To get started, create a folder named components
in the project's src
directory. Inside the newly created folder, create a file named Filter.jsx
and paste in the code below:
The code above defines an array called numbers
with ten numbers. It then defines a function called filterNumbersArray()
using the arrow function syntax. This function filters the numbers
array and returns an array containing odd numbers only. To achieve this, the function uses the JavaScript [filter()](<https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter>)
method, which takes a callback function as an argument. The callback function determines the filtering condition for each element in the numbers
array. In this case, the callback function uses the modulus operator (%) to check whether each number in the array is odd. If the number divided by two has a remainder that is not equal to zero (number % 2 !== 0
), it is considered an odd number, and it will be included in the filtered array. The filter()
method iterates through each element in the numbers
array while applying this condition and returns a new array containing only the values that satisfy the condition.
The filter()
function works well for performing simple filtering logic where you only need to extract specific values that satisfy a condition. You can also use this method if you prefer a concise and functional programming approach, as the filter()
function simplifies the code and makes it more readable. However, you should not use this method if you need to modify the original array in place. The filter()
function creates a new array and does not modify the original array.
Next, the code defines a piece of state to track the option selected by the user to either filter products based on their price or both the price and brand. The code then initializes a products
array that holds information about various products from different brands.
The filterByPriceHandler()
function is a handler function that takes value
as an argument, which represents a product from the products
array. This function checks whether the price
property of the product is greater than 800. If it is, the function returns true
, which indicates that the product can be included in the filtered array. Otherwise, it returns false
, indicating that the product should not be included in the filtered array.
The filterByPriceAndBrandHandler()
function is a handler function that filters the products
array based on the price
and brand
properties of each product. Similar to filterByPriceHandler()
, it takes an input value that represents a product. It then checks if the product's price
property value is greater than 800 and if the product's brand
property value is "Apple"
. If both conditions are met, the function returns true
, which indicates that the product can be included in the filtered array. Otherwise, it returns false
, indicating that the product should not be included in the filtered array.
You should consider using the filter()
method with a custom handler function if you:
- Have an array of custom objects and want to filter them based on one or more specific conditions
- Want to keep your code modular and reusable by encapsulating the filtering logic in a separate function
- Prefer a functional programming approach and want to leverage the expressive power of higher-order functions
However, it might not be best to use this method if your filtering logic is extremely complex and requires tight control over the filtering process. In such cases, a for()
loop with explicit conditional statements might provide better flexibility and clarity.
The code then renders the original arrays as well as the modified arrays on the user interface.
Using a For Loop
To demonstrate how to filter through records using a [for](<https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Loops_and_iteration#for_statement>)
loop, create a file named ForLoop.jsx
inside the src/components
directory and paste in the code below:
The code above defines an array of numbers called numbers
. The filterEvenNumbers()
function filters the numbers
array to return an array of even numbers. It initializes a new array called evenNumbers
and uses a for
loop to iterate through all the elements in the array. If an element is divisible by two (numbers[i] % 2 === 0
), it is pushed into the evenNumbers
array. The function finally returns the evenNumbers
array.
The filterEvenNumbers()
function does not contain a custom stop condition, which can be problematic in some scenarios. If the for
loop iterates over a large array, the time complexity of the loop would be O(n), where n is the number of elements in the array. As the size of the array increases, the time required for the loop to execute also increases proportionally. This implies that the larger the array, the longer it takes for the loop to complete. This might lead to slow performance and delays in rendering the results to the user.
The customFilterEvenNumbers()
function is similar to the filterEvenNumbers()
function, but it contains a custom stop condition. It initializes an empty array called evenNumbers
and iterates through the elements of the numbers
array to check for even numbers. However, when the evenNumbers
array length reaches three, the for
loop is stopped using the break
statement. This allows for faster, more optimized processing when the set condition is met, which, in this case, is the required number of even numbers.
The component finally renders the original array as well as the filtered arrays to the UI.
Using a for
loop might be best suited for use cases where:
- You need fine-grained control over the filtering process, such as skipping or jumping to specific elements based on certain conditions
- You need to perform complex operations on each element that go beyond simple filtering
If your use case requires simple filtering, it might be best to leverage the benefits of higher-order functions like filter()
.
Testing the application
To test that the code filters the records as expected, open the src/App.jsx
file and replace the existing code with the following:
The code above imports the newly created components. Next, open the src/main.jsx
file and delete the line that imports the src/index.css
file (import './index.css'
).
Next, run the command npm run dev
in the terminal to start a development server, and open http://localhost:5173 in the browser.
We should get the following result:
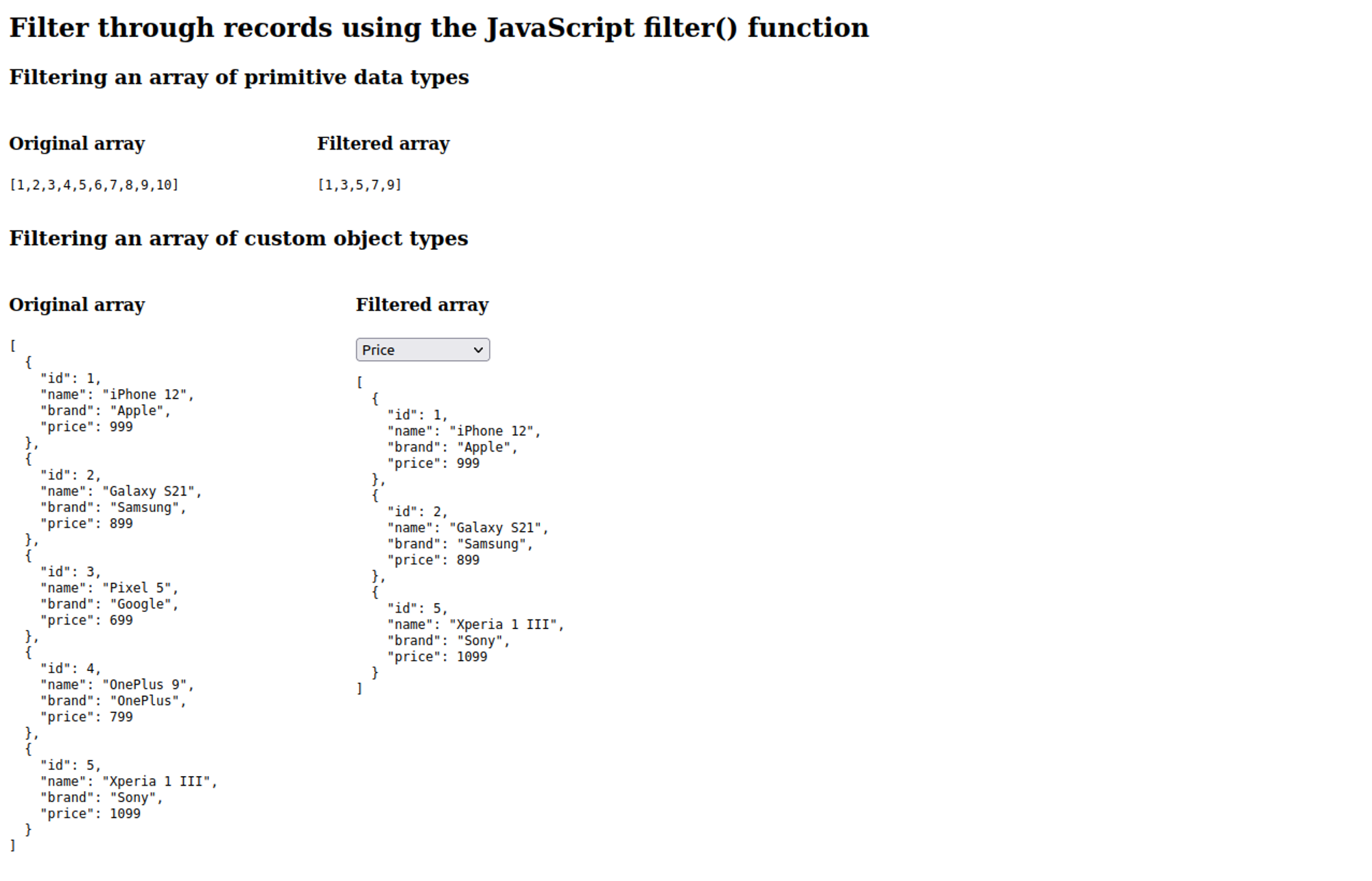
The above image shows the results of filtering an array of primitive data types as well as an array of custom object types. From the image, we can see that everything is working as expected. In the "Filtering an array of custom object types" section, we can choose whether to filter the array based on price alone or price and brand.
When we scroll down the page, we should see the following:
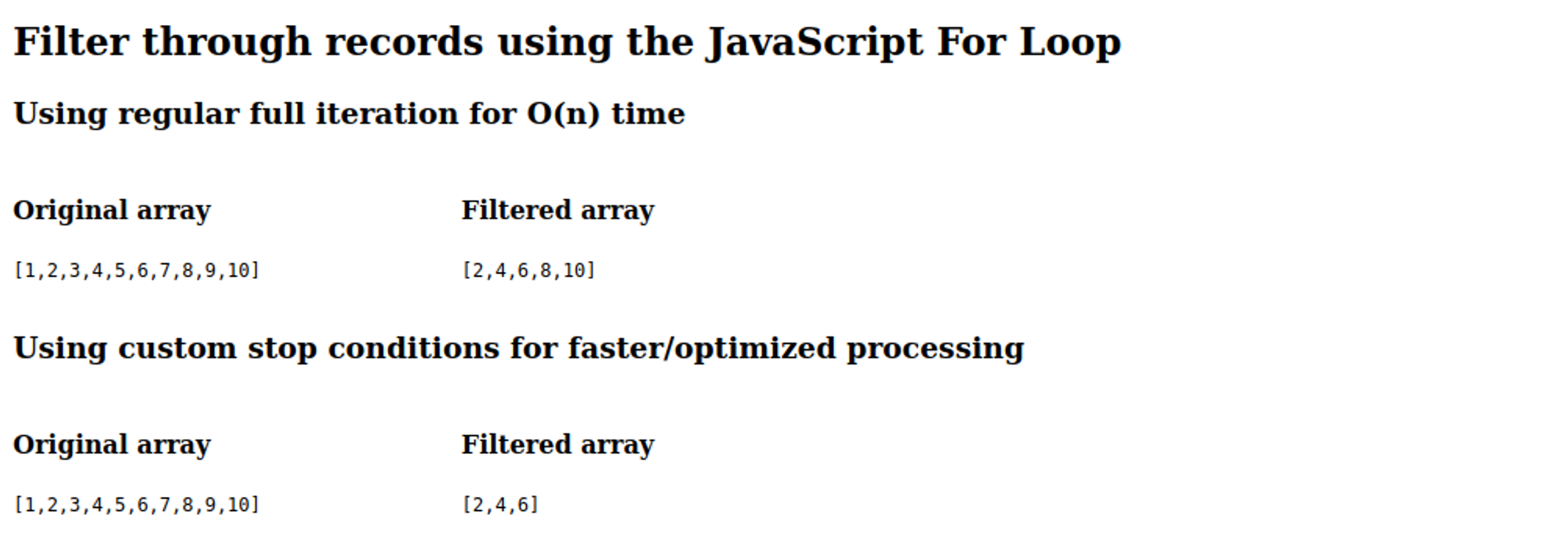
From the above screenshot, we can see that everything works as explained in the code.
Preprocessing data before rendering
Preprocessing data in React before rendering it in the user interface is a very common practice. There are several JavaScript functions, such as the [map()](<https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map>)
and [reduce()](<https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/reduce>)
functions, that help achieve this. These functions allow users to transform, aggregate, or manipulate data in a way that suits their application's needs before rendering it to the user interface.
The map()
function iterates over an array and returns a new array of transformed elements. This function allows us to preprocess data with operations such as formatting or extracting certain elements from each element in the array.
Here's an example of how to use the map()
function in React:
In the example above, the map()
function doubles each element in the numbers
array, creating a new array called newNumbers
.
Meanwhile, the reduce()
function aggregates array elements into a single value. It applies a function to each array element, taking the previously accumulated value and the current element as arguments. The final accumulated value represents the sum of all the elements in the array.
Here's an example of how to use the reduce()
function in React:
In the code above, the reduce()
function calculates the sum of the elements in the numbers
array. It takes an accumulator (accumulator
) and the current value (currentValue
) and adds them together. The initial value of the accumulator is set to zero.
By using the map()
and reduce()
functions in the React application, you have the flexibility to preprocess data according to your specific application requirements before displaying it in the user interface.
Filtering records in React can be a powerful way to display and understand data in depth. Tying it to a platform that allows you to quickly built React-based UIs will make it even easier to display and understand your data in depth, even for non-technical team members.
Introducing Airplane: rapidly build custom, React-based UIs
Airplane is the developer platform for building custom internal tools. You can transform scripts, queries, APIs, and more into powerful internal workflows and UIs within minutes. Airplane Views is Airplane’s React-based platform for building and customizing UIs for your teams. Airplane offers powerful built-in components and templates that make it simple to get started, reducing the time engineers spend on building complex internal tooling.
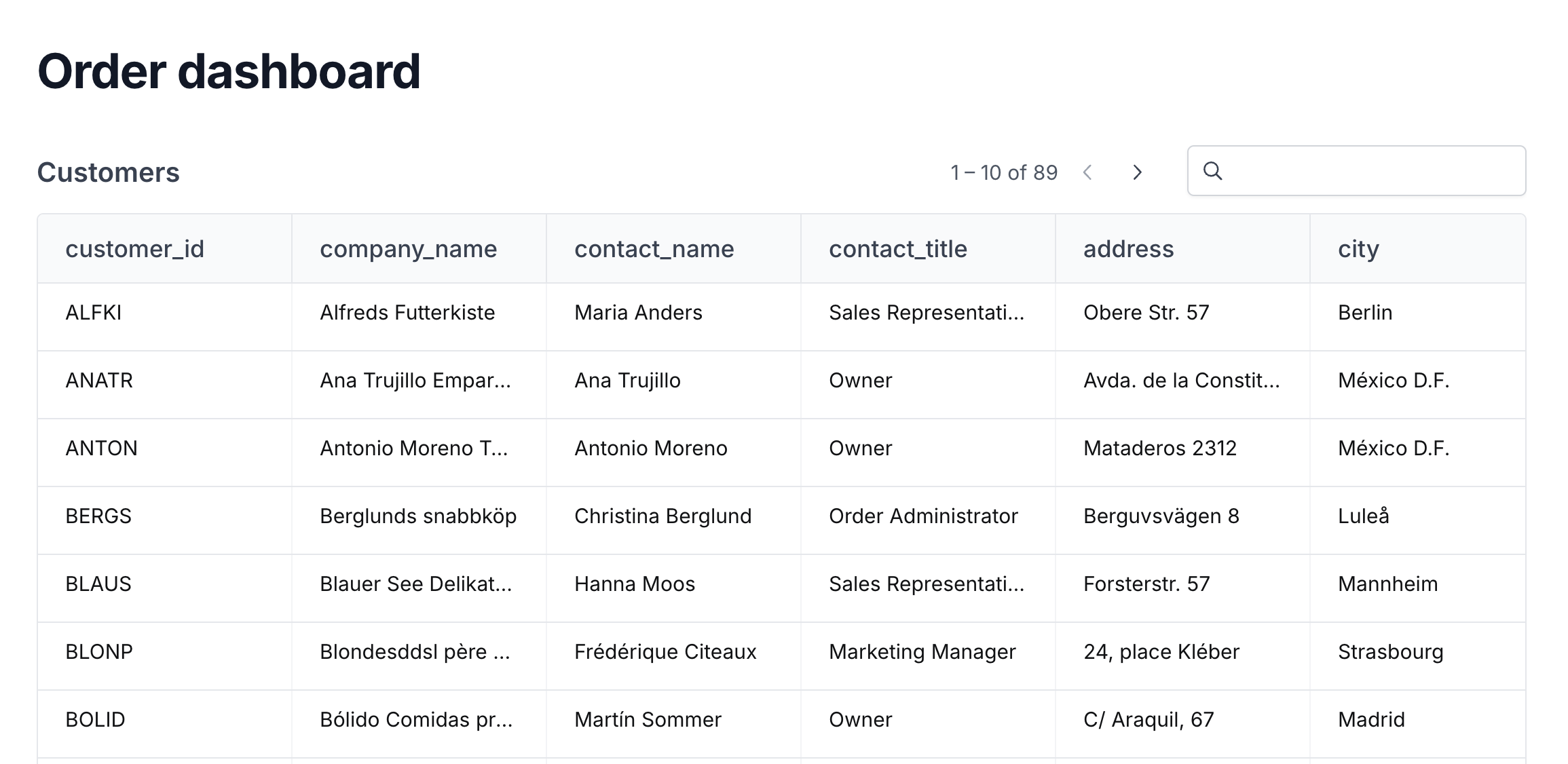
In addition to strong component and template offerings, Airplane has powerful out-of-the-box features, such as permissions settings, job scheduling, audit logs, and more. This ensures that everything you build and run in Airplane is safe, secure, and auditable.
To try it out and build powerful internal UIs quickly without compromising security, sign up for a free account or book a demo.
Author: Kevin Kimani
Kevin Kimani is a FullStack Web Developer specializing in React, Node.js and Django. He enjoys creating websites, writing technical articles and collaborating on open source projects.