What is a feature toggle?
A feature toggle, also known as a feature flag, is a mechanism used in the software development world to turn certain features of your project on and off remotely and without the need for deployment.
One of the main reasons to use feature flags inside your application is to roll out new changes to your product incrementally. For example, if you want only a subset of customers to be able to try out your app’s new search algorithm, you can wrap your code in a flag and turn the flag on just for select customers.
The advantages of feature flags go well beyond their use for deployments. In this article, we'll walk through the basics of feature toggles, some best practices when using them, and show you an example of how to manage feature flags with ease using Airplane, a developer tool for building internal apps.
Let's start off with some of the basics.
Feature toggles: the basics
To express a feature flag in its most simple form, you can just wrap your code behind a condition.
Here’s a basic example based on a personal case.
A while ago, I was teaching some students how to create a color flipper using JavaScript. We wrote a simple algorithm to generate a random hex color and display it on screen:
This is what the HTML page for this code looked like:
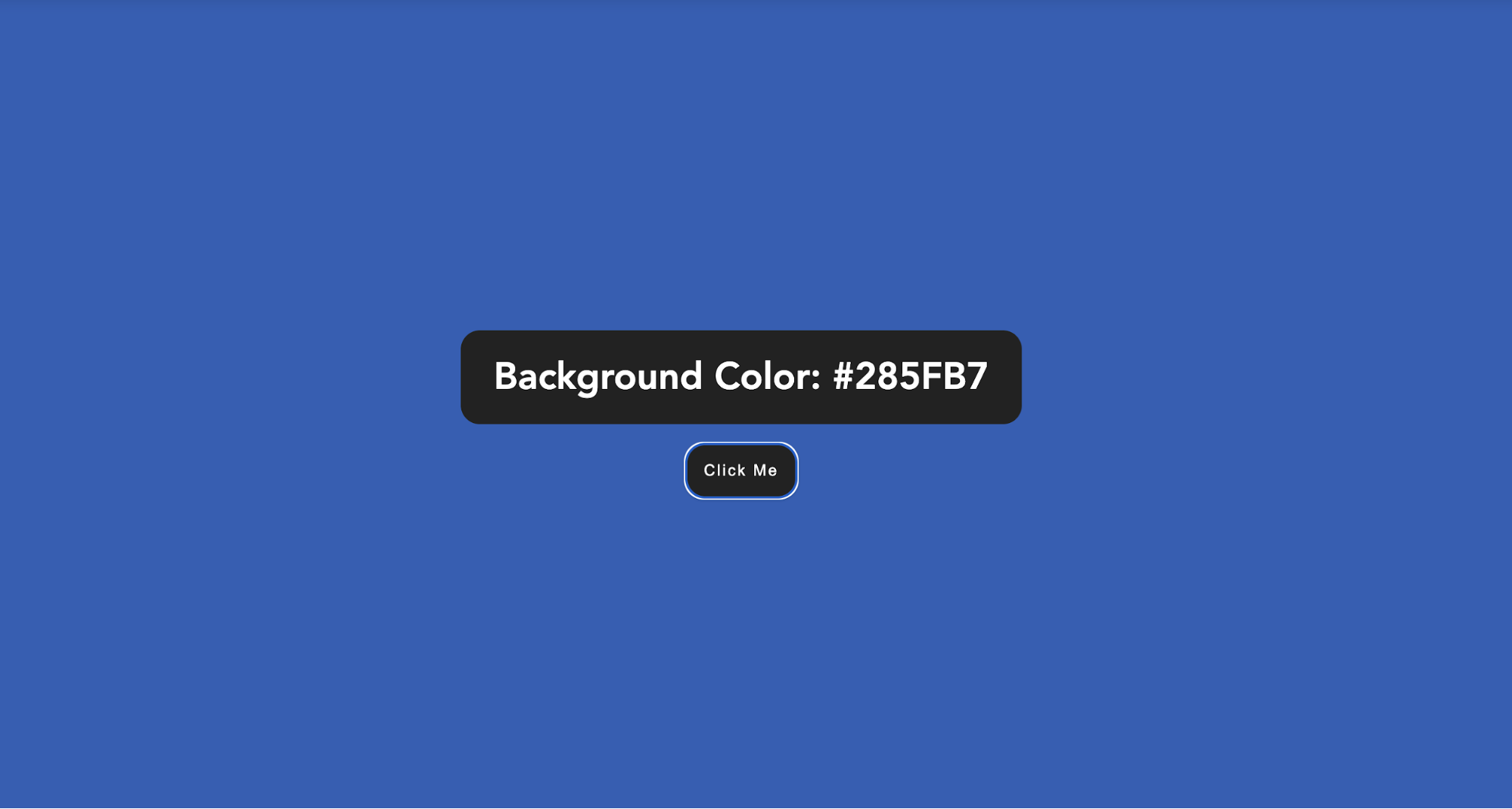
After the first example, some students asked me how to implement a second algorithm using RGB values this time, as well as how to implement a way to decide which users saw a particular algorithm.
This is a perfect case for a feature flag. It required me to trim my code by simply writing the following:
The final result looked like this:
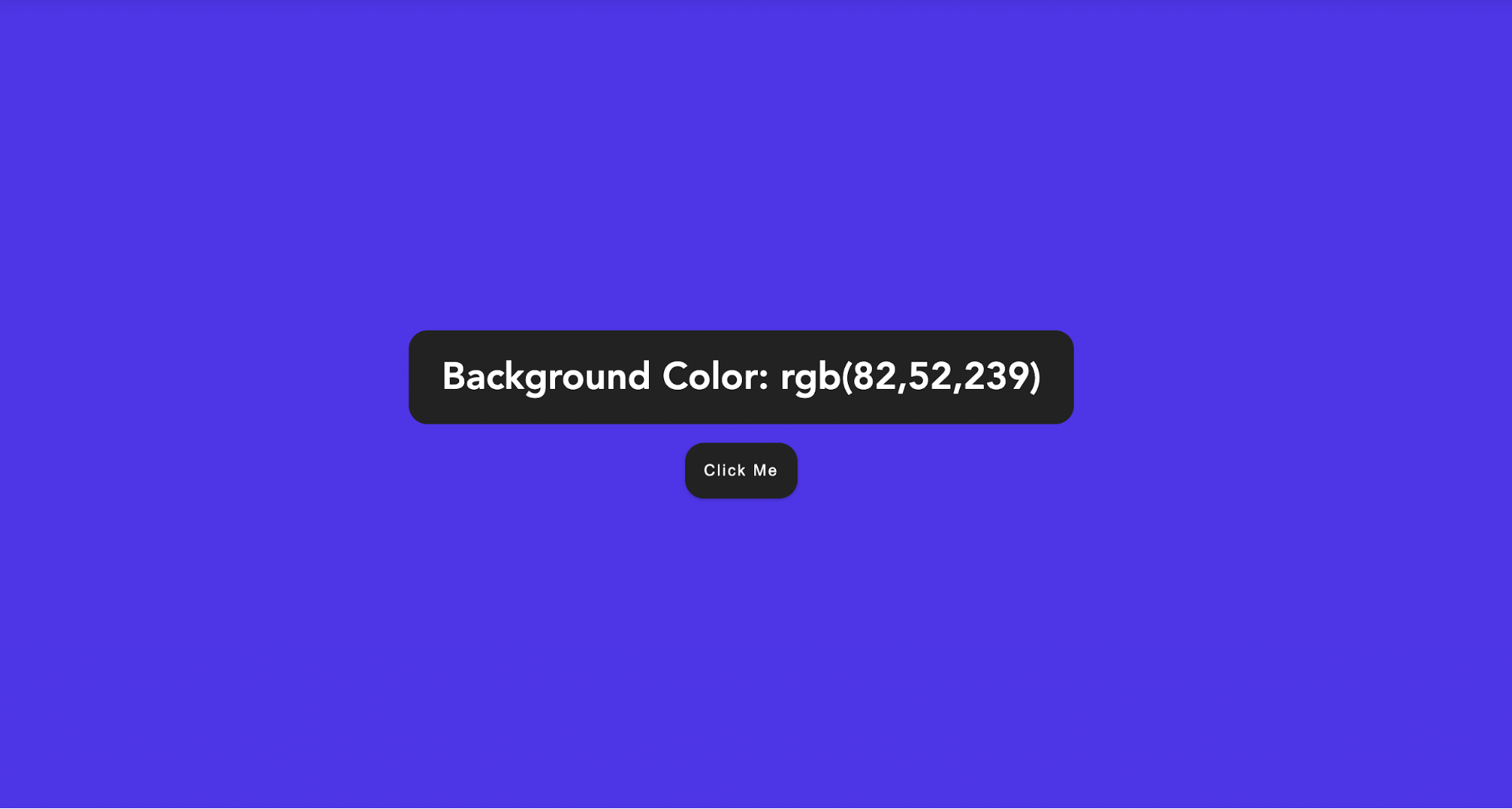
In this case, the users of the app were able to use the RGB algorithm only if the flag usesNewAlgorithm
was enabled, following a logic flow that looks like this:
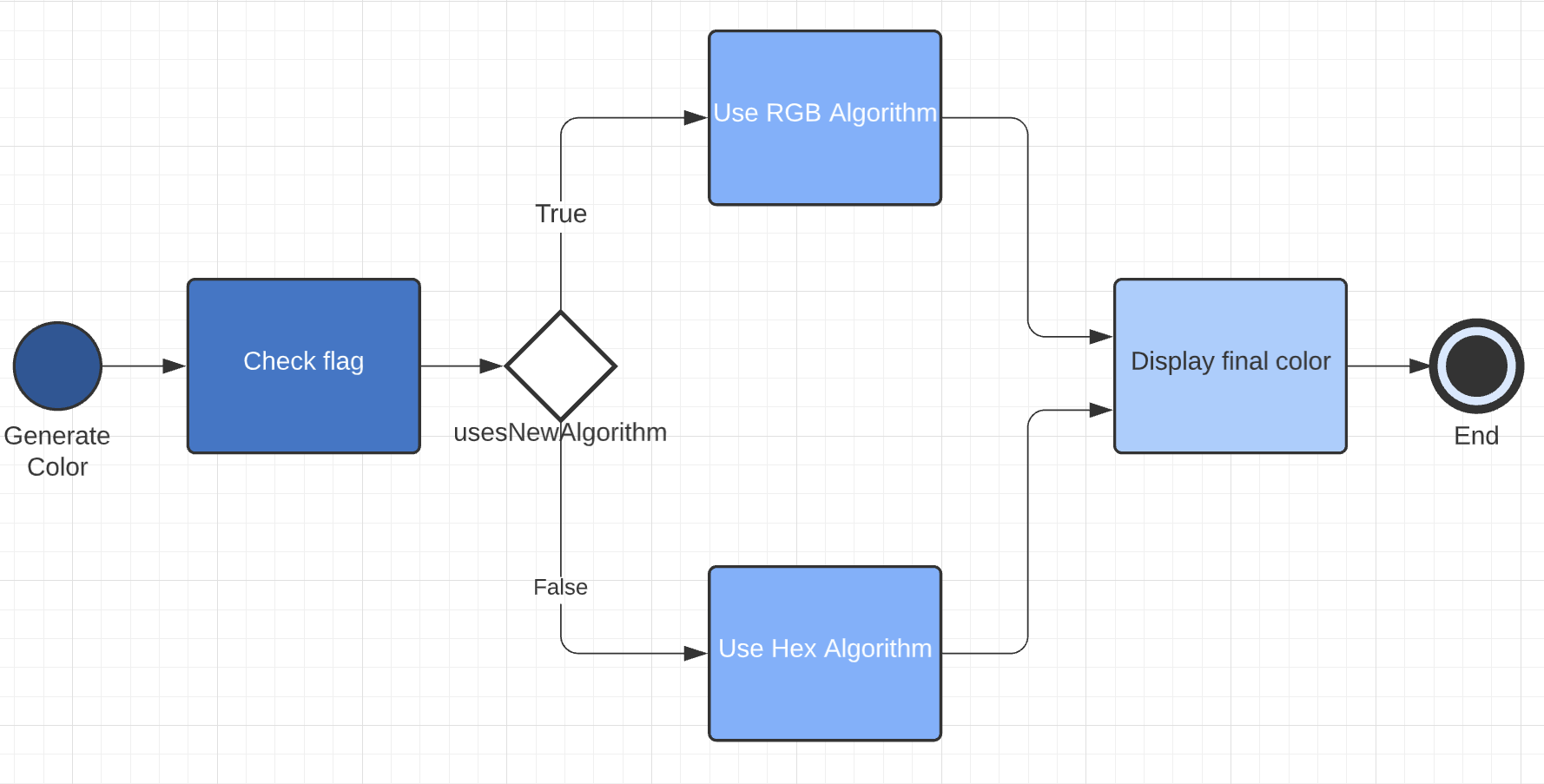
Apart from a simple example where you turn a functionality on or off, feature flags are well-suited for a series of testing and deployment scenarios. Consider a few additional use cases:
- Testing in production: Often times your products' testing and production environments differ. By introducing a feature flag in your code, you can slowly roll out new features in your production environment without risking compromising the entire product's integrity.
- A/B tests: Feature flags can also be used to run segmentations of your user base. You can wrap some of your code behind a flag for flow “X” and use a second flag for flow “Y”. By doing this, you can decide which users access which flows. You can also gather analytics based on their actions and compare and contrast the two flows.
- Canary releases: We know how important it is to test new features and any modifications to your the product. With a canary release approach, you can place your new feature behind a feature flag and turn the flag on for just a small set of users. You can then lean on that smaller set of users to provide feedback and clean up bugs before rolling the feature out more broadly.
Best practices for feature toggles
Now that we've walked through how using feature flags could be a great choice for your team, let’s take a look at some best practices to follow when working with them.
- Optimize the type of flag for your use case: Feature flags can be separated into two overarching types: short-term flags and permanent flags. The first are usually adopted to temporarily roll out a new feature or to test user preferences by running segmentation tests. Once these operations are done, the short-term flags are removed. Permanent flags, on the other hand, are designed to manage part of your code for an extended period of time. An example of this is managing feature levels for your app users, such as premium or basic subscriptions.
- Introduce flags in your design process: Including flags in discussions between product managers and developers early on could help save time later on in the developmental process. There can be a lot of complexity involved with implementing a flag in determining its suitability for the product. Instead of introducing flags later or wondering if you should've used them, we encourage you to include discussing them as a part of your design process.
- Use a standardized naming convention: Whatever category of flag you’re creating, having a standardized naming convention can help you track and manage your flags more easily. Without consistent naming, flags can become quite difficult to read, locate, and manage. We recommend using descriptive names with a capitalization standard, like camelCase or snake_case. This way, you won't have to spend additional time figuring out what specific flags actually do.
- Use default values: To avoid the risk of activating a feature accidentally, you'll want to make sure you have your flags' default values under control.
- Review your flags: We recommend reviewing your flags' usage at regular intervals of time. Otherwise, you risk amassing dozens of unused toggles over time, which could lead to a large technical debt for your product.
Depending on your scale, feature toggles may become quite difficult to manage. One option is outsourcing flag management. There are a number of third-party solutions you can use for this such as LaunchDarkly, Split, or CloudBees which provide a full suite of products to help manage features.
How Airplane can help manage feature flags
If you're looking to implement something quickly and easily while still using an enterprise-grade tool, Airplane is a great option to consider.
Airplane is a platform that helps engineers quickly turn code into secure, internal applications. You can use Airplane for things like runbook automation, scheduled operations, customer onboarding, and more.
While Airplane isn’t a dedicated feature flag platform like LaunchDarkly or Split, a lot of teams use Airplane for feature flags when they have simpler needs that don't require a heavyweight solution. For example, if all you really need is a way to turn on and off certain features for specific customers, Airplane will be the quickest way to implement this.
Later on, if you decide to adopt a dedicated feature management platform, you can use Airplane to hit their API and still use Airplane for much more granular permission management, audit logging, and approval flows.
Below, we walk through a quick tutorial on how to use Airplane to manage feature flags.
Feature toggle example in Airplane
Let's say you want to create a task to toggle feature flags at your company using Airplane. This might look something like: use an account ID to determine which account you want to update features for, have a set of features you can toggle, and then use a button to toggle them on or off.
We can create a SQL task in just a couple of minutes to accomplish this.
Create a new task
First, sign up for a free Airplane account and then click on New task on the side navigation bar. You have multiple options to create SQL, REST, or code-based tasks. Since we want to enable flags on our database, let's go ahead and select SQL for the task we're building.
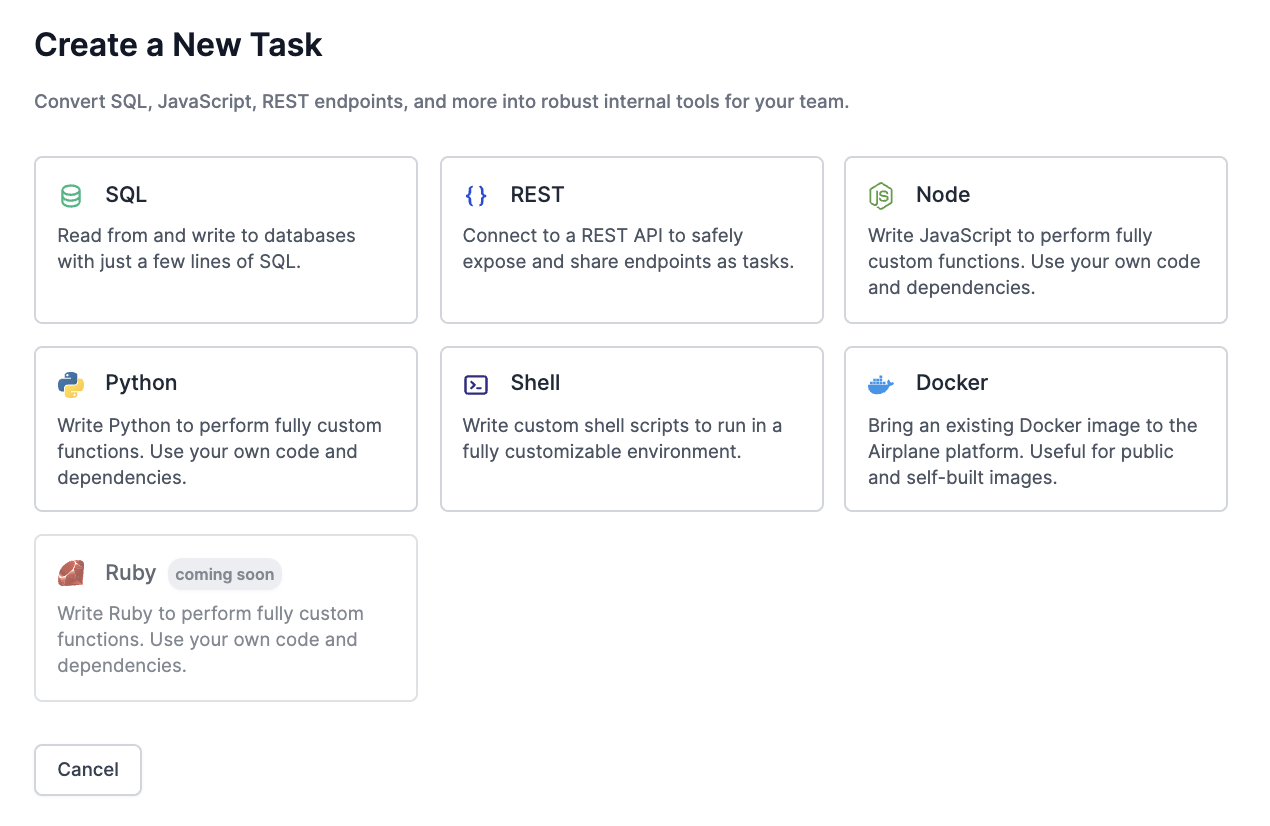
Define your task
Next, let's specify a Name and Description for our task so it's clear what the purpose of this task is.
- Name: Toggle feature flag for account
- Description: Turns an account's features on or off
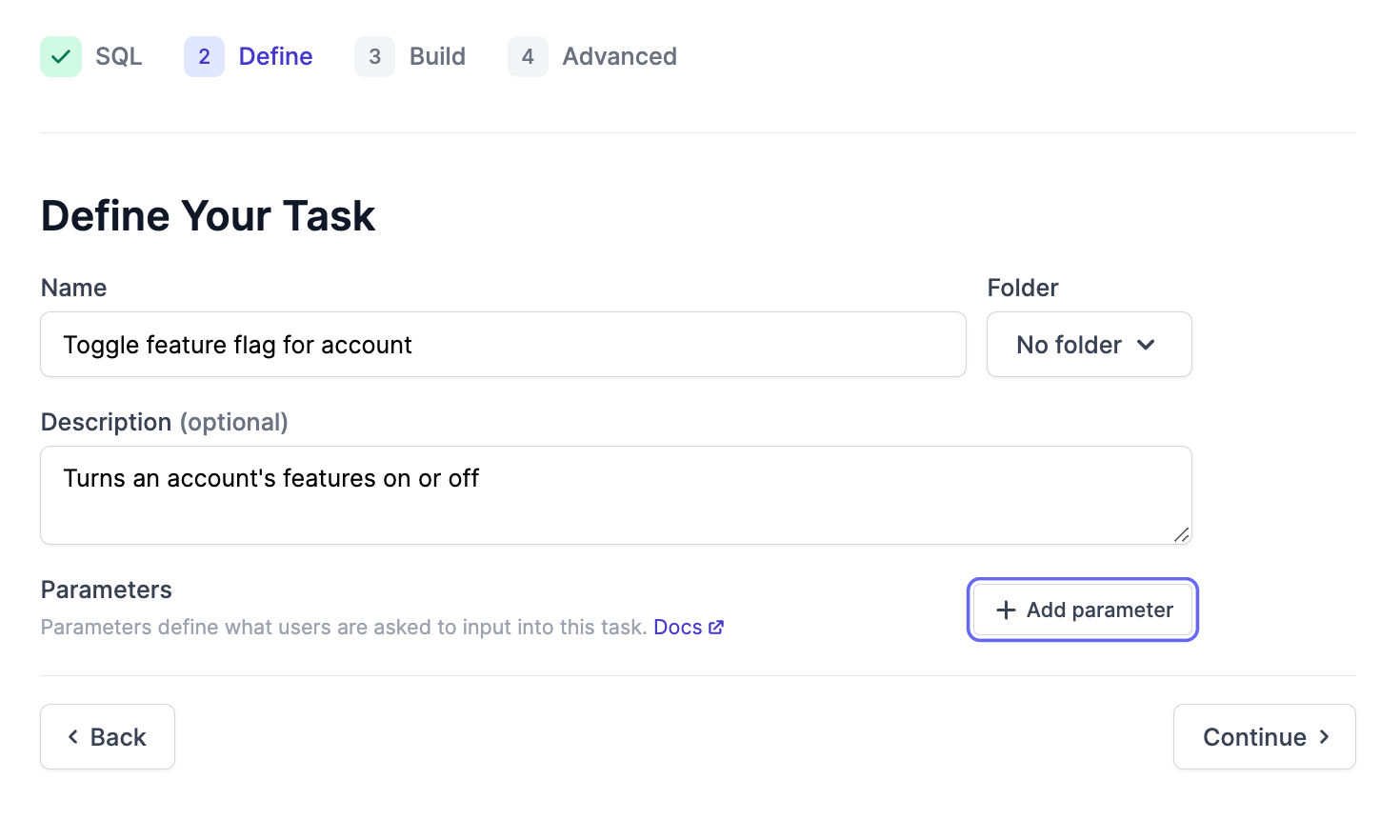
Add parameters
Now, we can add parameters for our task. We can add these by clicking the Add parameter button on the bottom right of the screen.
Inside the parameter-creation menu, you can set up different options based on your needs. You can specify a name and description for your param and you can also configure things like the parameter "Type" and whether or not the param should be required when using the task.
There are also a number of advanced settings which allow you to do thing such as dynamically control what values are allowed. You can read more on parameter types and config in our docs.
In this case, we want the user of the task to specify the following:
Account ID: The account we want to activate our flag for.
- Type = integer
Feature: The name of the feature/flag we want to activate.
- Type = short text
- For this param, let's require the user to pick from a list of options. Toggle on "Select options" and add labels for the features you're looking to enable/disable with this task. Ex: dark mode, JS templating, folders beta
On/off: A parameter to specify if the feature flag is turned on or off for the client.
- Type = boolean
This is what adding the Feature
parameter should look like:
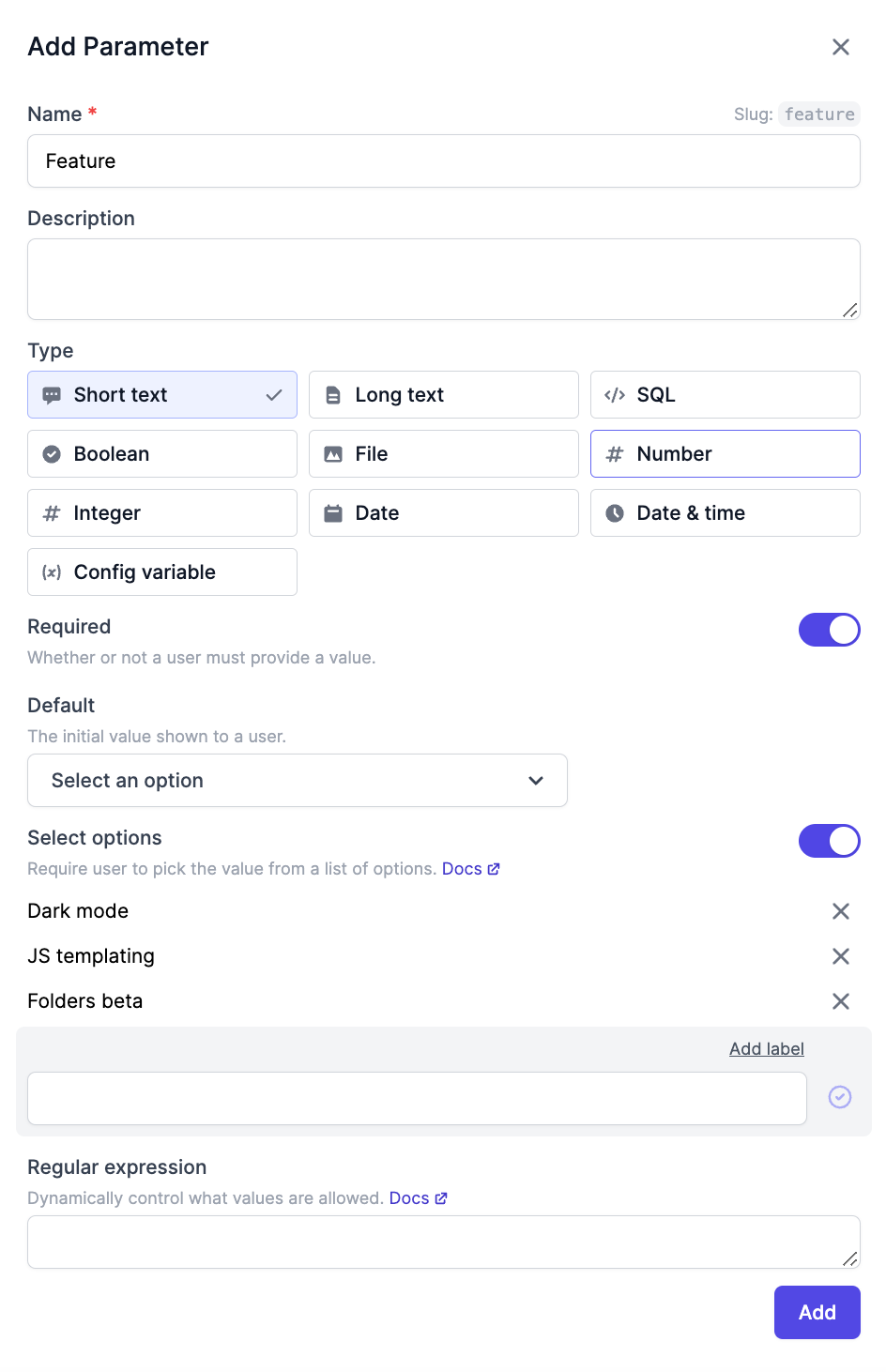
This is what adding the On/off
parameter using the boolean type should look like:
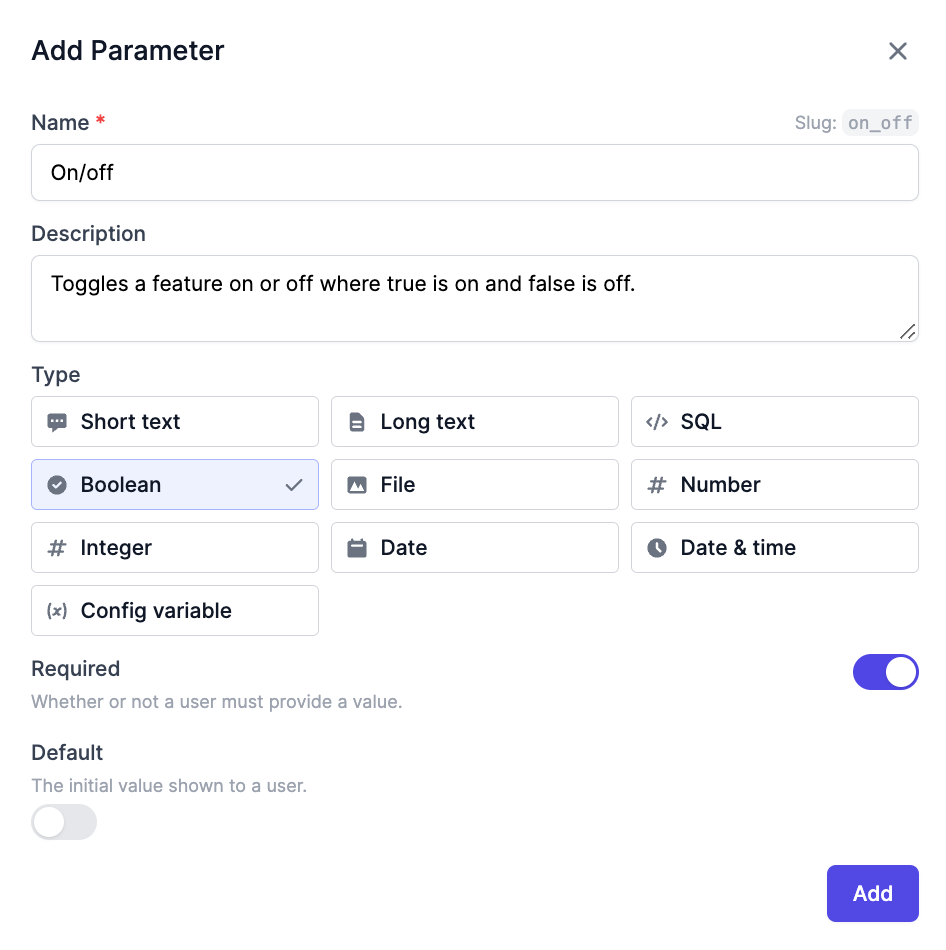
Once you've added all three parameters, you should see them listed on the task definition screen along with the original name and description we set.
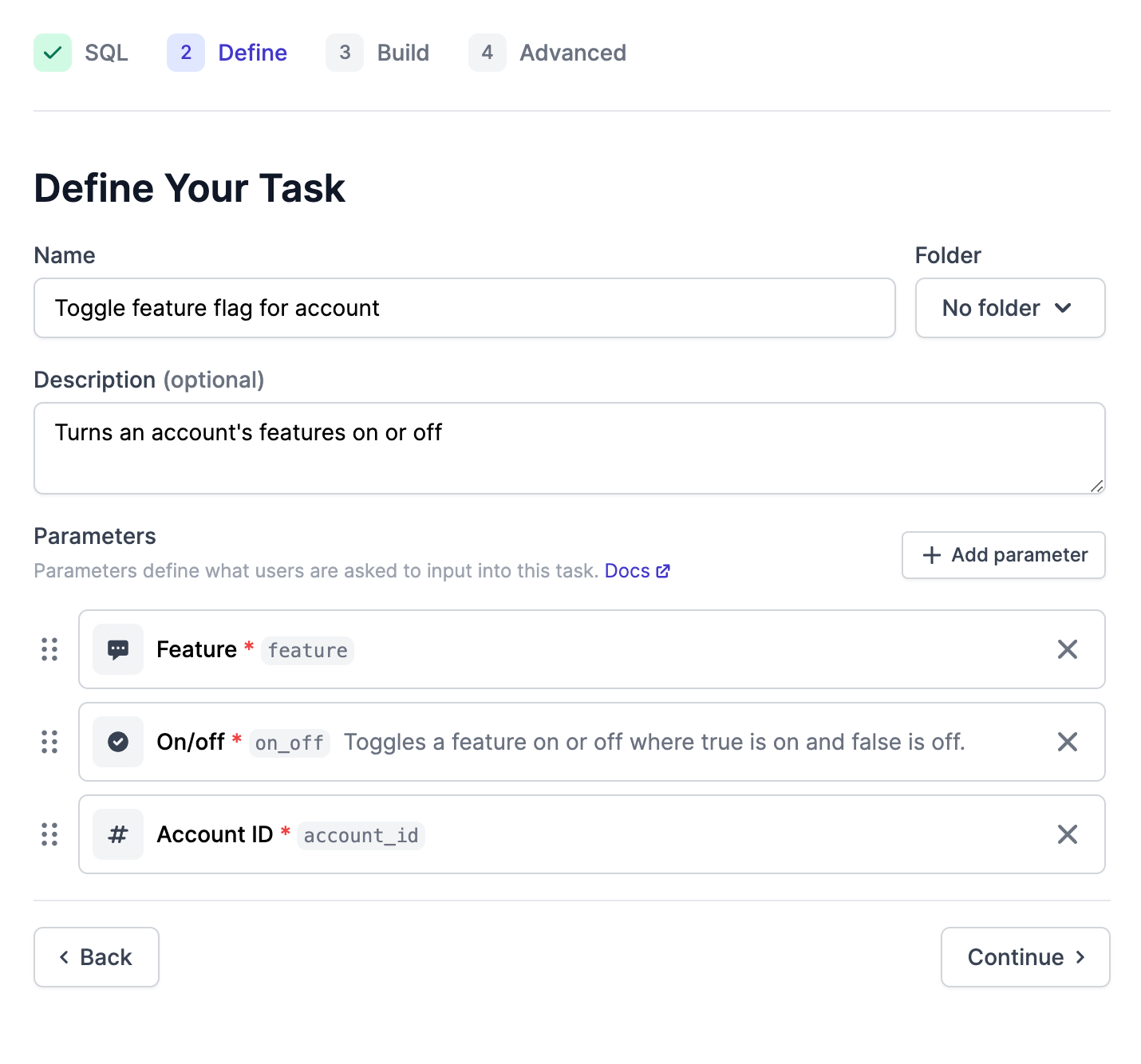
Next, we can click Continue and build our task by entering the SQL query we want the task to execute.
Build your task
You can enter one or more SQL queries into the query box on the task builder. Let's write something that updates our accounts
table to include a new feature that's being turned on or leave the active features list the same. This might look something like the below:
Inside of the query, we can refer to the params you created by prepending a colon (:
) before the param name.
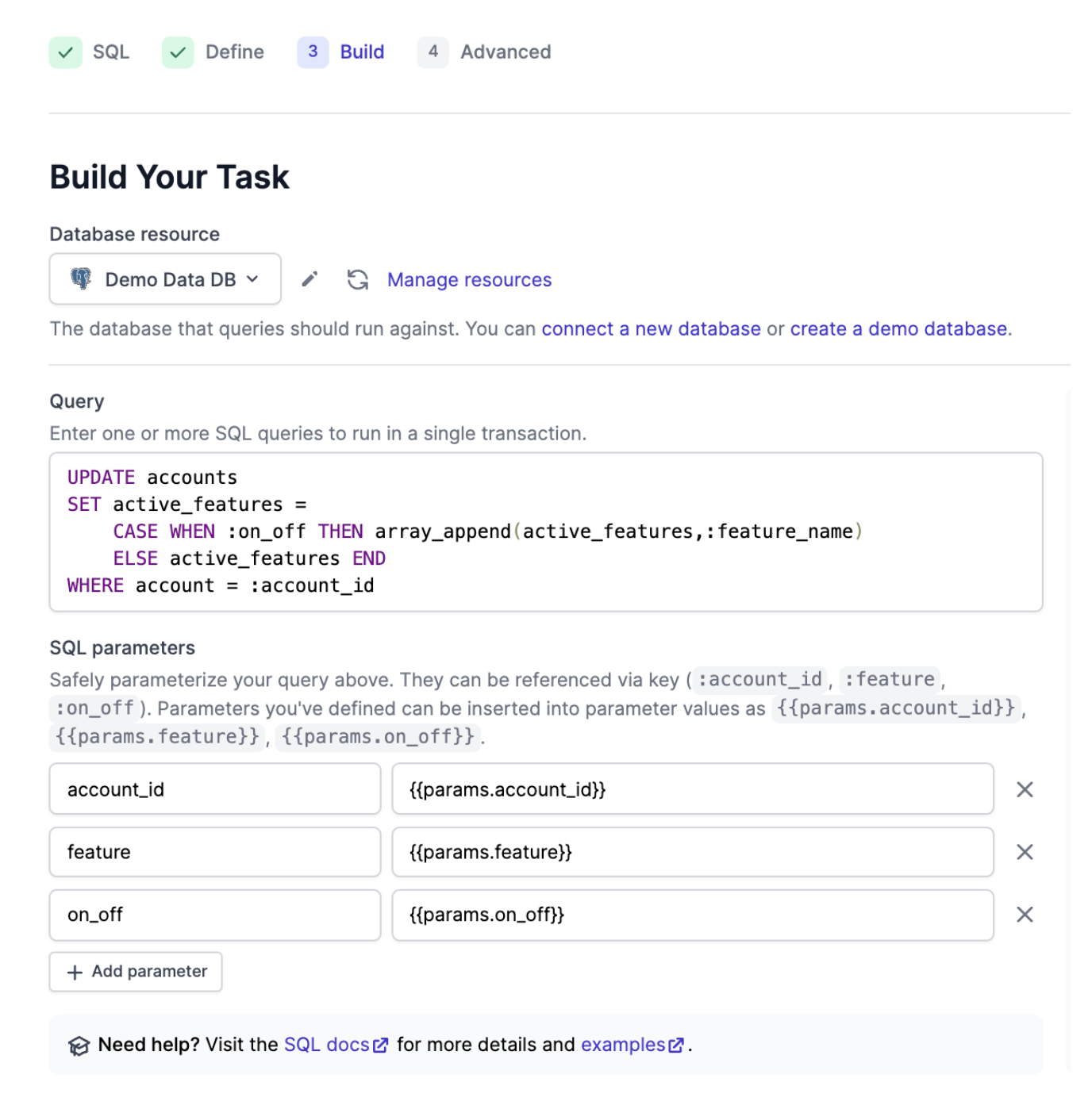
Now we're ready to create the task. Click Continue.
Advanced settings
The final step to building our task is to set Advanced Settings if we have any. These settings are used to manage who can access and execute this task. This is where we can allow full access to anyone on our team or set explicit permissions. Let's leave the permissions as "Team access" and click Create task.
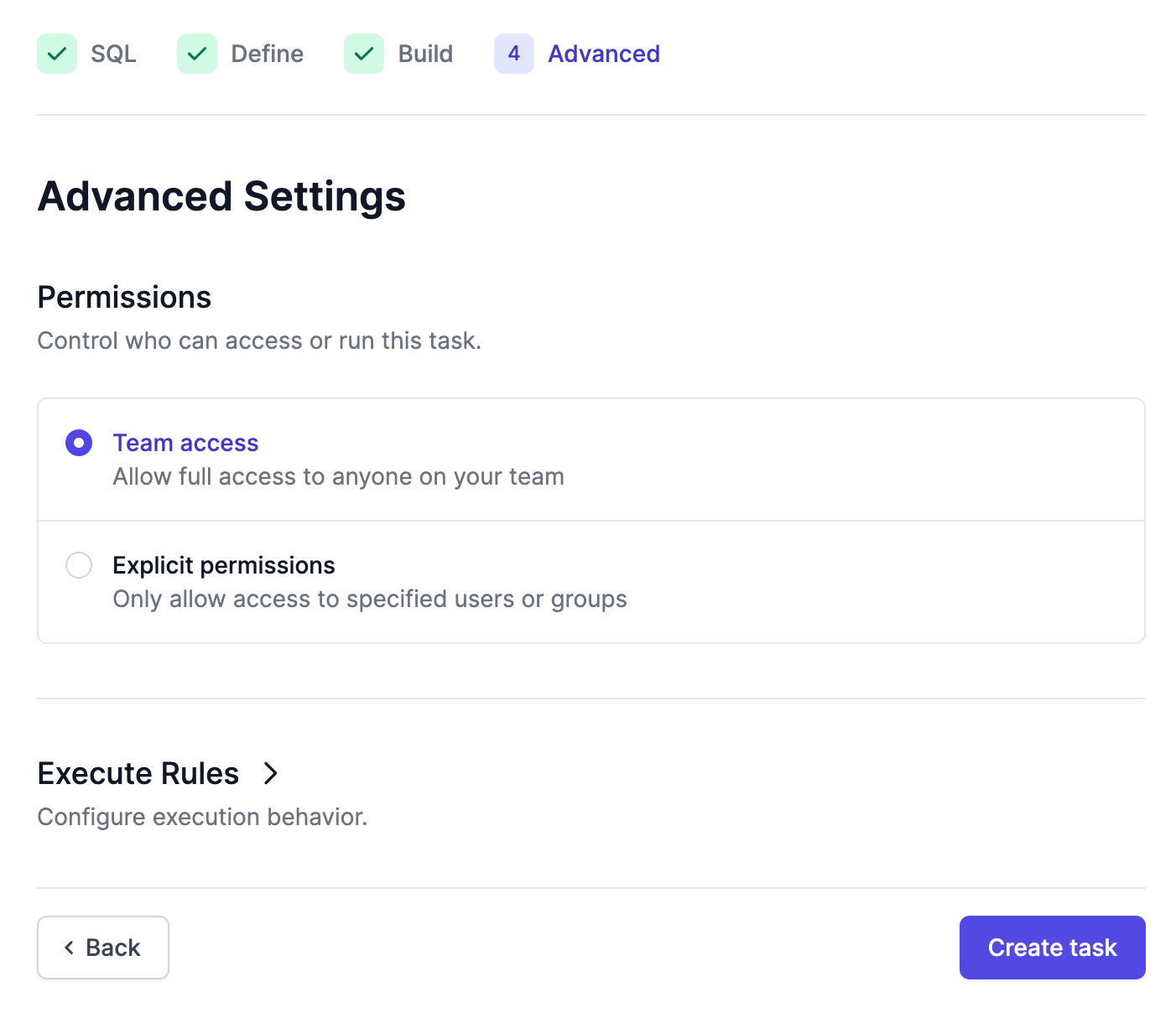
You'll now see the finished product - a simple, sharable web form representing the task we just created. Anyone with access can now enter the parameters and execute the task to manage features. That's it!
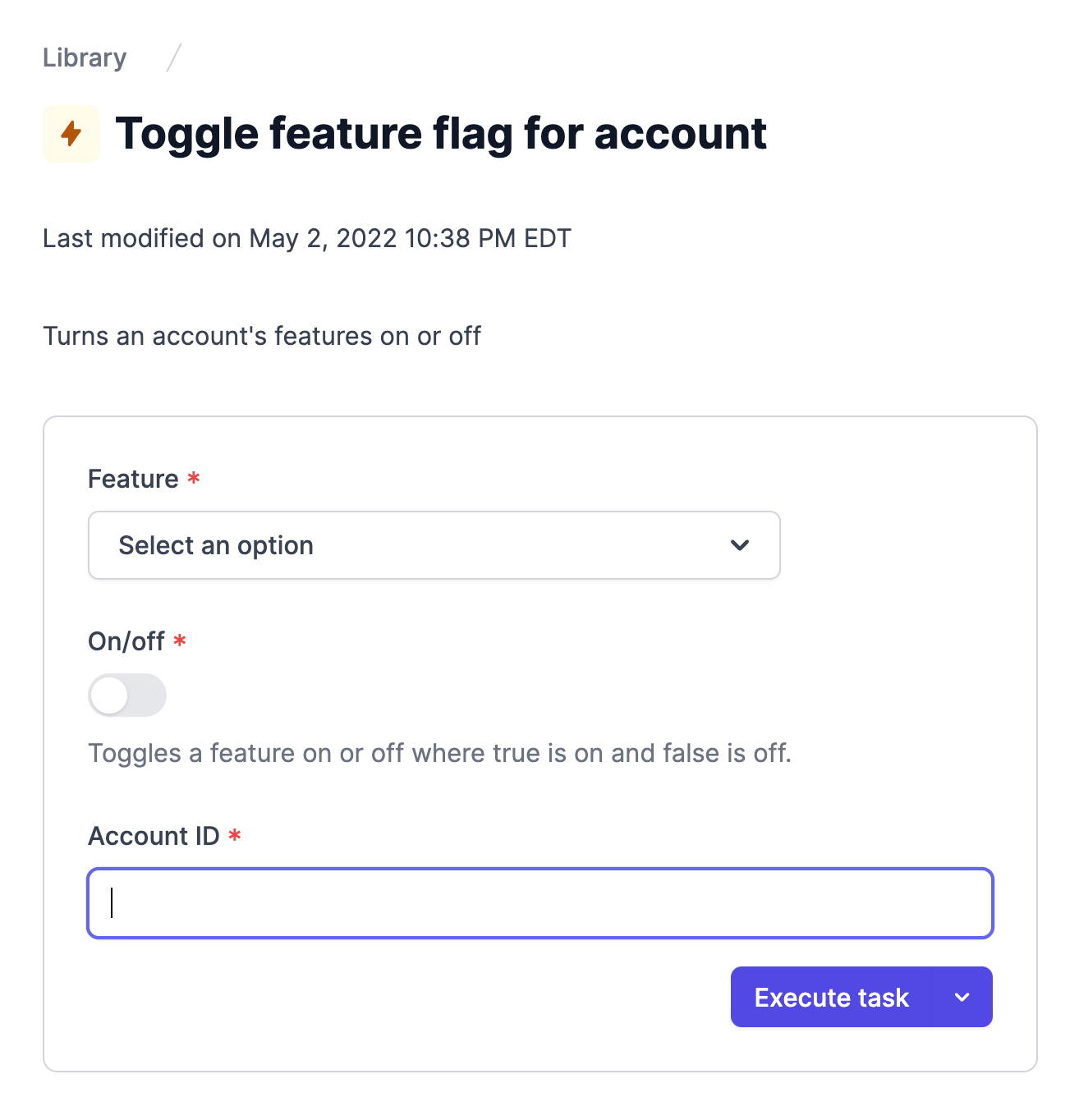
As you can see, you can create and manage feature flags in Airplane in just a matter of minutes. Airplane also provides a number of features you can layer on top of tasks such as group-based permissions, approval flows, schedules, notifications, and more.
Feature management is just one internal operation you can use Airplane for. You can learn more about Airplane use cases on our blog where we also post on topics like Runbook automation, Cron troubleshooting and Scheduling GitHub Actions. Visit our documentation to learn more about automating operational workflows and other capabilities.
This post was drafted by Piero Borrelli and edited by Madhura Kumar. Piero is an Italian software developer focused on web development and experienced in JavaScript. He's passionate about helping people achieve a better life by learning to code.